Introduction
This music responsible IQ light string is an application based on an individually addressable LED strip. It can turn into other different products if you want to. All you have to do is to change the outside wrapper. It's googd choice to be a decoration in your friends' party, Children's room, or date with candlelight dinner and romantic pas de deux. .. ^^
What you’ll need
Connection
You can connect different components together based on the diagram below:
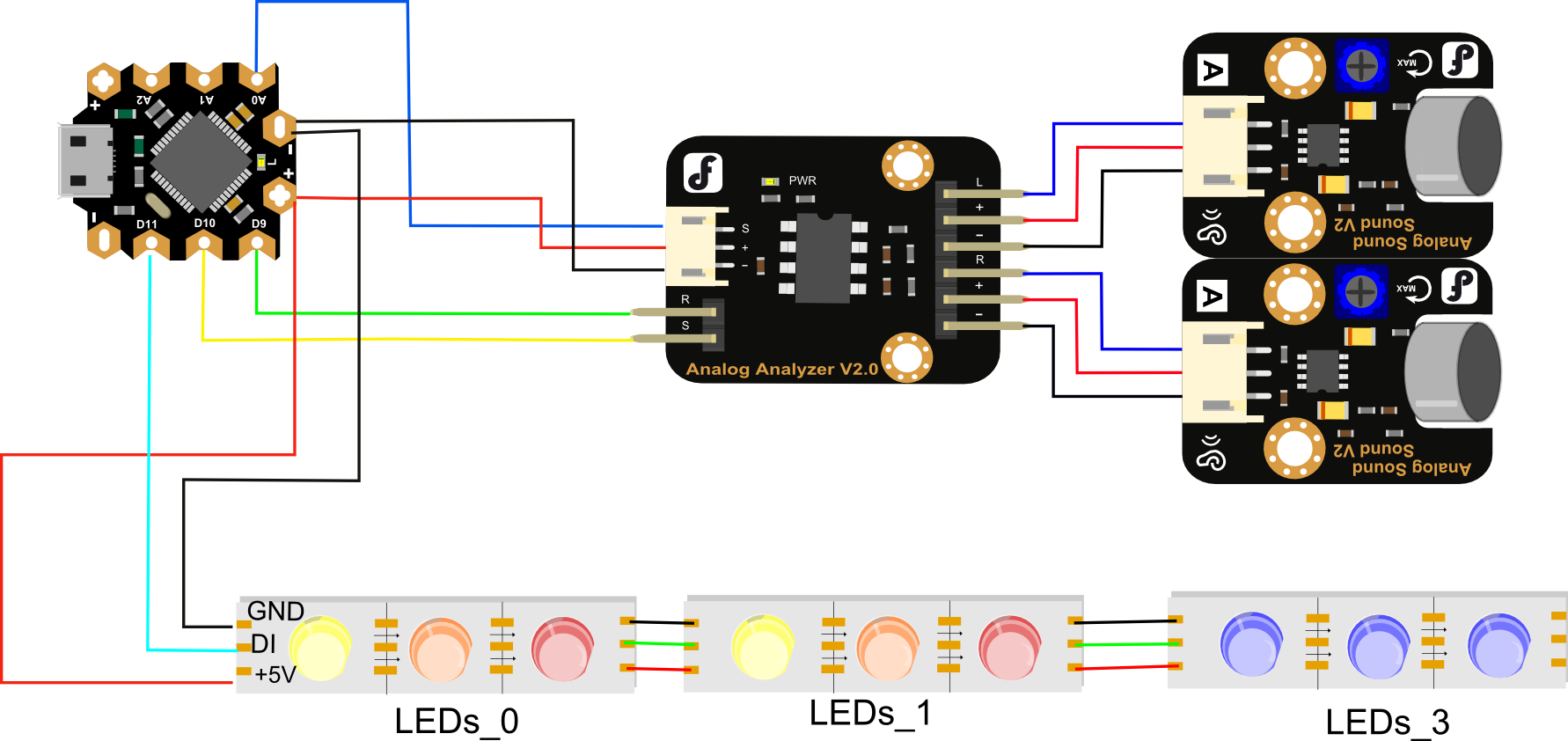
Note: pay attention to the direction of the strips when you cut and reconnect them, you may want to glue the soldering points just in case they get torn.
Installation
If it’s the first time you’re using Arduino, please install the Arduino IDE and the required drive. You can go to the following link for instructions:
Download the Library that is provided for you and import it to your Arduino. This will help you control your LEDs more easily.
Adafruit_NeoPixel
AudioAnalyzer
The CODE
Given the limited length of the led strip I have, I separated the led strip into 6 parts to display changes in various frequencies of the music. There are 7 frequencies that the Audio Analyzer can get, but you’ll find out some are not quite easy to use. Thus you’ll need to play around and see what works best for your own design. You can take a look at my code and start from it. Even more complicated patterns can be created, and I am quite looking forward to more designs .
/*music responsible led strip sample code
created by Yu Sun on 07/28/2015
*/
#include <Adafruit_NeoPixel.h>
#include <AudioAnalyzer.h>
#define PIN 11 //The signal pin connected with Arduino
#define LED_COUNT 34 //total number of leds in the strip
#define NOISE 120// noise that you want to chrop off
#define SEG 6 // how many parts you want to seperate the led strip into
Analyzer Audio = Analyzer(10,9,0);//Strobe pin ->10 RST pin ->9 Analog Pin ->0
Adafruit_NeoPixel leds = Adafruit_NeoPixel(LED_COUNT, PIN, NEO_GRB + NEO_KHZ800);
int FreqVal[7];//create an array to store the value of different freq
void setup()
{
Serial.begin(57600);
Audio.Init();
leds.begin(); // Call this to start up the LED strip.
clearLEDs(); // This function, defined below, turns all LEDs off...
leds.show(); // ...but the LEDs don't actually update until you call this.
}
void loop(){
Audio.ReadFreq(FreqVal);
for (int i = 0;i<7;i++){
FreqVal[i]=constrain(FreqVal[i],NOISE,1023);
FreqVal[i]=map( FreqVal[i],NOISE,1023,0,255);
Serial.print(FreqVal[i]);//used for debugging and Freq choosing
Serial.print(" ");
}
int j;
//assign different values for different parts of the led strip
for (j=0;j<LED_COUNT;j++){
if(0<=j && j<=LED_COUNT/7)
{
set(j,FreqVal[1]);// set the color of led
leds.show();
delay(1.5);// to make the led transit color more naturally
}
else if((LED_COUNT/SEG)<=j && j<(LED_COUNT/SEG*2))
{
set(j,FreqVal[1]);
leds.show();
delay(1.5);
}
else if((LED_COUNT/SEG*2)<=j&& j<(LED_COUNT/SEG*3)){
set(j,FreqVal[3]);
leds.show();
delay(1.5);
}
else if((LED_COUNT/SEG*3)<=j&& j<(LED_COUNT/SEG*4)){
set(j,FreqVal[4]);
leds.show();
delay(1.5);
}
else if((LED_COUNT/SEG*4)<=j&& j<(LED_COUNT/SEG*5)){
set(j,FreqVal[3]);
leds.show();
delay(1.5);
}
else{
set(j,FreqVal[2]);
leds.show();
delay(1.5);
}
}
}
//the following function set the led color based on its position and freq value
void set(byte position, int value){
if(0<=position&& position<LED_COUNT/SEG){
leds.setPixelColor(position,leds.Color(position*15+value*15,position*5+value*6,0));
}
else if(LED_COUNT/SEG<=position && position<LED_COUNT/SEG*2){
leds.setPixelColor(position,leds.Color(position*5+value*5,value+position*2,0));
}
else if(LED_COUNT/SEG*2<=position&& position<LED_COUNT/SEG*3){
leds.setPixelColor(position,leds.Color(value*5+position*3,value*4+position*2,0));
}
else if(LED_COUNT/SEG*3<=position&& position<LED_COUNT/SEG*4){
leds.setPixelColor(position,leds.Color(0,value*8+position,position*0.96+value*2));
}
else if(LED_COUNT/SEG*4<=position&& position<LED_COUNT/SEG*5){
leds.setPixelColor(position,leds.Color(0,(value*2+position*0.2)*3,(value+position*0.5)*3));
}
else{
leds.setPixelColor(position,leds.Color(value*0.4+position*0.8,value*0.3,value*0.5+position*0.2));
}
}
void clearLEDs()
{
for (int i=0; i<LED_COUNT; i++)
{
leds.setPixelColor(i, 0);
}
}
That’s it! Enjoy the sights and sounds!