DFPlayer mini : Unable to begin

Hi every one,
I'm trying to make this exemple code working but my ESP32 keep saying me that it was unable to begin the serial communication.
Serial monitor output
I'm using an ESP32 DOIT Devkit v1, I've tried to put the resistor as a pull down for the TX line as see on another tutorial, same for the RX line.
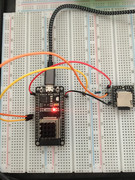
The content of my SD (yes I tried to remove the .Spotlight folder but it doesn't help, I've also run "dot_clean" to get rid of all other macOS dotfiles)
My code
Thanks
I'm trying to make this exemple code working but my ESP32 keep saying me that it was unable to begin the serial communication.
Serial monitor output
Code: Select all
This is my setupDFRobot DFPlayer Mini Demo
Initializing DFPlayer ... (May take 3~5 seconds)
Unable to begin:
1.Please recheck the connection!
2.Please insert the SD card!
I'm using an ESP32 DOIT Devkit v1, I've tried to put the resistor as a pull down for the TX line as see on another tutorial, same for the RX line.
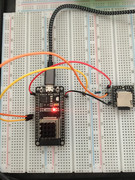
The content of my SD (yes I tried to remove the .Spotlight folder but it doesn't help, I've also run "dot_clean" to get rid of all other macOS dotfiles)
Code: Select all
➜ ~ ls -la /Volumes/UNTITLED
total 6912
[email protected] 1 sebastien staff 32768 Jul 3 15:22 .
[email protected] 4 root wheel 136 Jul 3 15:19 ..
drwxrwxrwx 1 sebastien staff 32768 Jul 3 15:21 .Spotlight-V100
-rwxrwxrwx 1 sebastien staff 3456451 Jul 3 13:58 001.mp3
My code
Code: Select all
I really can't see what I'm doing wrong, if someone can help me to get this running it would be amazing#include "Arduino.h"
#include <HardwareSerial.h>
#include "DFRobotDFPlayerMini.h"
#define RX2 16
#define TX2 17
HardwareSerial MySerial(1);
DFRobotDFPlayerMini myDFPlayer;
void printDetail(uint8_t type, int value);
void setup()
{
MySerial.begin(9600, SERIAL_8N1, RX2, TX2);
Serial.begin(115200);
Serial.println();
Serial.println(F("DFRobot DFPlayer Mini Demo"));
Serial.println(F("Initializing DFPlayer ... (May take 3~5 seconds)"));
if (!myDFPlayer.begin(MySerial)) {
Serial.println(F("Unable to begin:"));
Serial.println(F("1.Please recheck the connection!"));
Serial.println(F("2.Please insert the SD card!"));
while(true);
}
Serial.println(F("DFPlayer Mini online."));
myDFPlayer.setTimeOut(500); //Set serial communictaion time out 500ms
//----Set volume----
myDFPlayer.volume(10); //Set volume value (0~30).
myDFPlayer.volumeUp(); //Volume Up
myDFPlayer.volumeDown(); //Volume Down
//----Set different EQ----
myDFPlayer.EQ(DFPLAYER_EQ_NORMAL);
// myDFPlayer.EQ(DFPLAYER_EQ_POP);
// myDFPlayer.EQ(DFPLAYER_EQ_ROCK);
// myDFPlayer.EQ(DFPLAYER_EQ_JAZZ);
// myDFPlayer.EQ(DFPLAYER_EQ_CLASSIC);
// myDFPlayer.EQ(DFPLAYER_EQ_BASS);
//----Set device we use SD as default----
// myDFPlayer.outputDevice(DFPLAYER_DEVICE_U_DISK);
myDFPlayer.outputDevice(DFPLAYER_DEVICE_SD);
// myDFPlayer.outputDevice(DFPLAYER_DEVICE_AUX);
// myDFPlayer.outputDevice(DFPLAYER_DEVICE_SLEEP);
// myDFPlayer.outputDevice(DFPLAYER_DEVICE_FLASH);
//----Mp3 control----
// myDFPlayer.sleep(); //sleep
// myDFPlayer.reset(); //Reset the module
// myDFPlayer.enableDAC(); //Enable On-chip DAC
// myDFPlayer.disableDAC(); //Disable On-chip DAC
// myDFPlayer.outputSetting(true, 15); //output setting, enable the output and set the gain to 15
//----Mp3 play----
myDFPlayer.next(); //Play next mp3
delay(1000);
myDFPlayer.previous(); //Play previous mp3
delay(1000);
myDFPlayer.play(1); //Play the first mp3
delay(1000);
myDFPlayer.loop(1); //Loop the first mp3
delay(1000);
myDFPlayer.pause(); //pause the mp3
delay(1000);
myDFPlayer.start(); //start the mp3 from the pause
delay(1000);
myDFPlayer.playFolder(15, 4); //play specific mp3 in SD:/15/004.mp3; Folder Name(1~99); File Name(1~255)
delay(1000);
myDFPlayer.enableLoopAll(); //loop all mp3 files.
delay(1000);
myDFPlayer.disableLoopAll(); //stop loop all mp3 files.
delay(1000);
myDFPlayer.playMp3Folder(4); //play specific mp3 in SD:/MP3/0004.mp3; File Name(0~65535)
delay(1000);
myDFPlayer.advertise(3); //advertise specific mp3 in SD:/ADVERT/0003.mp3; File Name(0~65535)
delay(1000);
myDFPlayer.stopAdvertise(); //stop advertise
delay(1000);
myDFPlayer.playLargeFolder(2, 999); //play specific mp3 in SD:/02/004.mp3; Folder Name(1~10); File Name(1~1000)
delay(1000);
myDFPlayer.loopFolder(5); //loop all mp3 files in folder SD:/05.
delay(1000);
myDFPlayer.randomAll(); //Random play all the mp3.
delay(1000);
myDFPlayer.enableLoop(); //enable loop.
delay(1000);
myDFPlayer.disableLoop(); //disable loop.
delay(1000);
//----Read imformation----
Serial.println(myDFPlayer.readState()); //read mp3 state
Serial.println(myDFPlayer.readVolume()); //read current volume
Serial.println(myDFPlayer.readEQ()); //read EQ setting
Serial.println(myDFPlayer.readFileCounts()); //read all file counts in SD card
Serial.println(myDFPlayer.readCurrentFileNumber()); //read current play file number
Serial.println(myDFPlayer.readFileCountsInFolder(3)); //read file counts in folder SD:/03
}
void loop()
{
static unsigned long timer = millis();
if (millis() - timer > 3000) {
timer = millis();
myDFPlayer.next(); //Play next mp3 every 3 second.
}
if (myDFPlayer.available()) {
printDetail(myDFPlayer.readType(), myDFPlayer.read()); //Print the detail message from DFPlayer to handle different errors and states.
}
}
void printDetail(uint8_t type, int value){
switch (type) {
case TimeOut:
Serial.println(F("Time Out!"));
break;
case WrongStack:
Serial.println(F("Stack Wrong!"));
break;
case DFPlayerCardInserted:
Serial.println(F("Card Inserted!"));
break;
case DFPlayerCardRemoved:
Serial.println(F("Card Removed!"));
break;
case DFPlayerCardOnline:
Serial.println(F("Card Online!"));
break;
case DFPlayerUSBInserted:
Serial.println("USB Inserted!");
break;
case DFPlayerUSBRemoved:
Serial.println("USB Removed!");
break;
case DFPlayerPlayFinished:
Serial.print(F("Number:"));
Serial.print(value);
Serial.println(F(" Play Finished!"));
break;
case DFPlayerError:
Serial.print(F("DFPlayerError:"));
switch (value) {
case Busy:
Serial.println(F("Card not found"));
break;
case Sleeping:
Serial.println(F("Sleeping"));
break;
case SerialWrongStack:
Serial.println(F("Get Wrong Stack"));
break;
case CheckSumNotMatch:
Serial.println(F("Check Sum Not Match"));
break;
case FileIndexOut:
Serial.println(F("File Index Out of Bound"));
break;
case FileMismatch:
Serial.println(F("Cannot Find File"));
break;
case Advertise:
Serial.println(F("In Advertise"));
break;
default:
break;
}
break;
default:
break;
}
}
Thanks
2022-01-21 18:15:10 I am trying to play music using Arduino Uno and nano serial Rx and tx pin but I don't get success to achieve this
got many type error like
"DFRobot DFPlayer Mini Demo
Initializing DFPlayer ... (May take 3~5 seconds)
Unable to begin:
1.Please recheck the connection!
2.Please insert the SD card! "
while we connect rx and tx pin to 1 and 0 respectively
rather than if i connect those pin to pin 11 and 10 its working
what is the reason behind this?
i don't know
please solve this and show me a right way and give me solution...
code is listed down..
teamexperientialetc
got many type error like
"DFRobot DFPlayer Mini Demo
Initializing DFPlayer ... (May take 3~5 seconds)
Unable to begin:
1.Please recheck the connection!
2.Please insert the SD card! "
while we connect rx and tx pin to 1 and 0 respectively
rather than if i connect those pin to pin 11 and 10 its working
what is the reason behind this?
i don't know
please solve this and show me a right way and give me solution...
code is listed down..
Code: Select all
waiting for responce to solve issues #include "Arduino.h"
#include "SoftwareSerial.h"
#include "DFRobotDFPlayerMini.h"
SoftwareSerial mySoftwareSerial (0 , 1); // RX, TX
DFRobotDFPlayerMini myDFPlayer;
void printDetail();
void setup()
{ mySoftwareSerial.begin(9600);
Serial.begin(115200);
Serial.println();
Serial.println(F("DFRobot DFPlayer Mini Demo"));
Serial.println(F("Initializing DFPlayer ... (May take 3~5 seconds)"));
if (!myDFPlayer.begin(mySoftwareSerial)) { //Use softwareSerial to communicate with mp3.
Serial.println(F("Unable to begin:"));
Serial.println(F("1.Please recheck the connection!"));
Serial.println(F("2.Please insert the SD card!"));
while (true);
}
Serial.println(F("DFPlayer Mini online."));
myDFPlayer.volume(30); //Set volume value. From 0 to 30
myDFPlayer.play(1); //Play the first mp3
}
void loop()
{
static unsigned long timer = millis();
if (millis() - timer > 3000) {
timer = millis();
myDFPlayer.next(); //Play next mp3 every 3 second.
}
if (myDFPlayer.available()) {
printDetail(myDFPlayer.readType(), myDFPlayer.read()); //Print the detail message from DFPlayer to handle different errors and states.
}
}
void printDetail(uint8_t type, int value) {
switch (type) {
case TimeOut:
Serial.println(F("Time Out!"));
break;
case WrongStack:
Serial.println(F("Stack Wrong!"));
break;
case DFPlayerCardInserted:
Serial.println(F("Card Inserted!"));
break;
case DFPlayerCardRemoved:
Serial.println(F("Card Removed!"));
break;
case DFPlayerCardOnline:
Serial.println(F("Card Online!"));
break;
case DFPlayerPlayFinished:
Serial.print(F("Number:"));
Serial.print(value);
Serial.println(F(" Play Finished!"));
break;
case DFPlayerError:
Serial.print(F("DFPlayerError:"));
switch (value) {
case Busy:
Serial.println(F("Card not found"));
break;
case Sleeping:
Serial.println(F("Sleeping"));
break;
case SerialWrongStack:
Serial.println(F("Get Wrong Stack"));
break;
case CheckSumNotMatch:
Serial.println(F("Check Sum Not Match"));
break;
case FileIndexOut:
Serial.println(F("File Index Out of Bound"));
break;
case FileMismatch:
Serial.println(F("Cannot Find File"));
break;
case Advertise:
Serial.println(F("In Advertise"));
break;
default:
break;
}
break;
default:
break;
}
}

2021-05-24 17:59:02 I skilled the identical issue. I observed that the solder joint at the TX pin changed into bridged to the SD card holder, efficiently bridging the pin to ground. Try a continuity check among the TX and ground. If they're related you've got got a terrible joint.I changed into capable of get rid of the extra solder and it labored quality after that. Its a medium issue soldering task.
You can go to here:https://thumzap.com/
calowoj196
You can go to here:https://thumzap.com/

2020-09-19 11:24:29 If its still relevant.....
I experienced the same issue. I found that the solder joint on the TX pin was bridged to the SD card holder, effectively bridging the pin to ground. Try a continuity test between the TX and ground. If they are connected you have a bad joint.
I was able to remove the excess solder and it worked fine after that. It's a medium difficulty soldering task.
mdisen1
I experienced the same issue. I found that the solder joint on the TX pin was bridged to the SD card holder, effectively bridging the pin to ground. Try a continuity test between the TX and ground. If they are connected you have a bad joint.
I was able to remove the excess solder and it worked fine after that. It's a medium difficulty soldering task.
