The objective of this micropython tutorial is to explain how to parse a JSON string with MicroPython running on the ESP32.
Introduction
The objective of this post is to explain how to parse a JSON string with MicroPython running on the ESP32. If you need help setting MicroPython on the ESP32, please check this previous post for a detailed guide. The guide also explains how to connect to the Python prompt.
In order to parse a JSON string, we will use the MicroPython uJSON library.
Since we are going to use the command line for testing the code, we will need a tool to help us compress the JSON content in a single line, so we can easily paste it. So, we will use this website, which can receive a JSON string and compress it to a single line. Then, we can past it to Putty just by copying the JSON and right clicking on the terminal window.
The code
After connecting to the Python prompt, we are ready to start coding. So, the first thing we need to do is importing the uJSON module. Just type the expression bellow and hit enter.
1 import ujson
After that, we should have access to the ujson object, which makes available a method called loads. This method receives as input the JSON string and returns an object corresponding to the parsed JSON [1]. We will start with a simple JSON structure, which is the one shown bellow.
1 {
2 "name":"John"
3 },
After compressing it to a one liner, we get the JSON string shown bellow.
1 {"name":"John"},
So, we will now pass this string as input of the previously mentioned loads method. Note that we will enclose the string between “”” in each side, in order to escape the quotes from the JSON structure. We will store the object in a variable called parsed.
1 parsed = ujson.loads("""{"name":"John"}""")
Now, we will confirm that we have the content of the JSON correctly in our returning object. So we will print it. Additionally, we will print the type of the object with the type function.
1 print (parsed)
2 print (type(parsed))
After running the whole previous code, we should get an output similar to figure 1. Note that the type of our object with the parsed content is a Python dictionary, making it perfect for accessing the content in a key-value style.
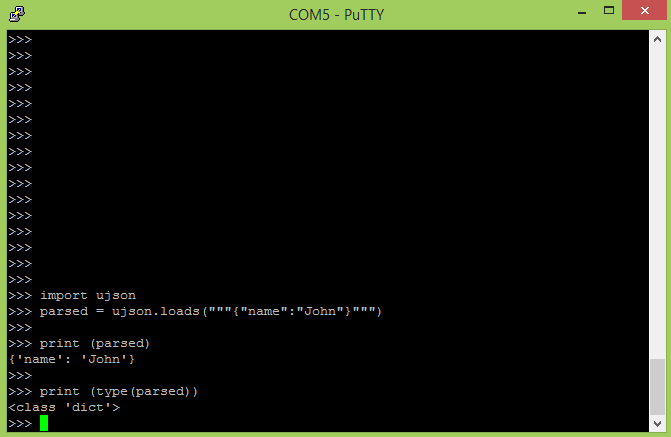
Figure 1 – Parsing the JSON string.
Now, we will access the value for the key equal to “name”. It should return “John”. In order to access the value for that key in the dictionary, send the command bellow. Note that it is like accessing an array value but instead of using an index, we use a key, in the format of a string.
1 print(parsed["name"])
You should get an output similar to figure 2. Note that the name “John” is printed on the console.
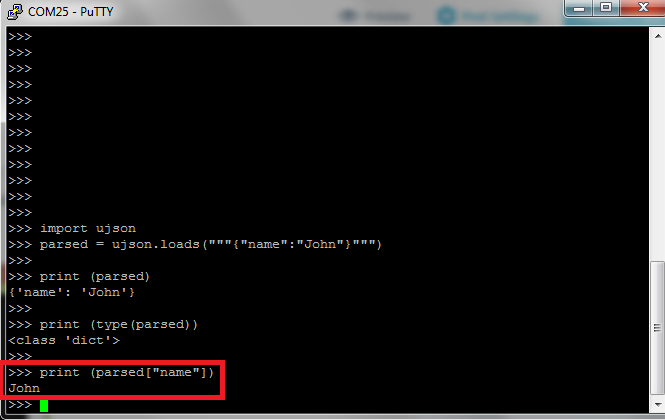
Figure 2 – Accessing the parsed values of the dictionary object.
To finalize our example, we will now parse a more complex structure, as shown bellow. This could represent, for example, a message sent from an IoT device.
1 {
2 "device":"temperature",
3 "id":543,
4 "values":[1,2,3]
5 }
After compressing, we get the following:
1 {"device":"temperature","id":543,"values":[1,2,3]}
So, we will now parse it and print all the keys existing in our JSON structure. We will also print the type of the “values” structure, to understand how it is mapped by the parser.
1 parsed = ujson.loads("""{"device":"temperature","id":543,"values":[1,2,3]}""")
2 print (parsed["device"])
3 print (parsed["id"])
4 print (parsed["values"])
5
6 print(type(parsed["values"]))
You should get a result similar to figure 3. As can be seen, all the values for each key are printed correctly. In the case of the “values” key, the structure inside the dictionary is a list, rather than a string representation of the values. Naturally, this is much better since we can operate over those values with all the functions available for lists, making them easier to manipulate.
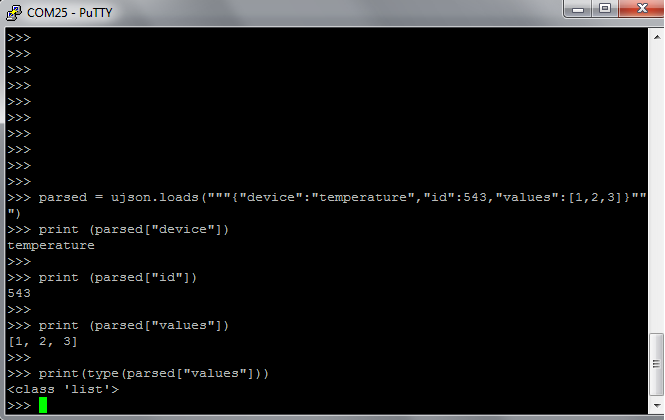
Figure 3 – Result of the JSON content parsed.
NOTE: This article is written by Nuno Santos who is an kindly Electronics and Computers Engineer. live in Lisbon, Portugal. you could check the original article here.
He had written many useful tutorials and projects about ESP32, ESP8266, If you are interested, you could check his blog to know more.