The objective of this MicroPython Tutorial is to explain how to perform HTTP POST requests using MicroPython and the urequests library. This was tested on both the ESP32 and the ESP8266.
Introduction
The objective of this MicroPython Tutorial is to explain how to perform HTTP POST requests using MicroPython and the requests library. This was tested on both the ESP32 and the ESP8266. The prints shown here are from the tests performed on the ESP8266.
We are going to send the HTTP POST request to a fake online testing REST API. The main website can be seen here. It supports multiple routes and we are going to use the /posts one. Note however that the name of the route to be used doesn’t have anything to do with the POST method we are going to use. In this case, a post corresponds to a dummy object representing a written post of a user in, for example, a website. On the other hand, POST is the HTTP method we are going to use.
Naturally, to follow this tutorial, the device needs to be previously connected to the Internet, so it can send the HTTP request. Please check this previous post on how to connect a device running MicroPython to a WiFi network. If you want to automate the connection after the device boots, check this other post. In my case, my MicroPython setup automatically connects the device to a WiFi network after the booting procedure.
Important: At the time of writing, the version of MicroPython used had urequests included by default. So, we would only need to import it, without performing any additional procedure. Note however that this may change and newer versions of MicroPython may no longer include it by default and require additional configuration procedures.
The code
The first thing we are going to do is importing the urequests module, to access the function needed to perform the HTTP POST request.
1 import urequests
Then we are going to send the request by calling the postfunction of the urequests module. This function receives as input the URL where we want to make the HTTP post request. It can also receive additional arguments in the form of a list of key – argument, since as can be seen by the function definition it has a **kw argument defined in the prototype. You can read more about the **kwargs in this very good article.
Since the post function calls the request function in it’s body, we can check that one of the additional arguments that we can pass is the data parameter. This corresponds to the body of our HTTP POST request.
Since this is a simple example to learn how to use the function, we will just send a string of data as body and we will not specify any particular content-type. Naturally, in a real case scenario, we would wan’t to specify a content-type and respect its format on the body of our request.
Note that the URL corresponds to the /posts route of the fake online REST api website mentioned in the introductory section.
Note that this function call will return an object of class Response, which we stored in a variable, so we can process the results of the HTTP request later.
Figure 1 shows the result of this same POST request, performed using Postman. As can be seen, we will receive an answer indicating a new resource was created (a post object with ID 101), independently of the content of our request.
If we keep sending requests, the answer will always be the same, since we are dealing with a fake test API. This is why we didn’t bother specifying the content-type or a request body that would make much sense.
If you need help with sending HTTP POST requests with Postman, please consult this video.
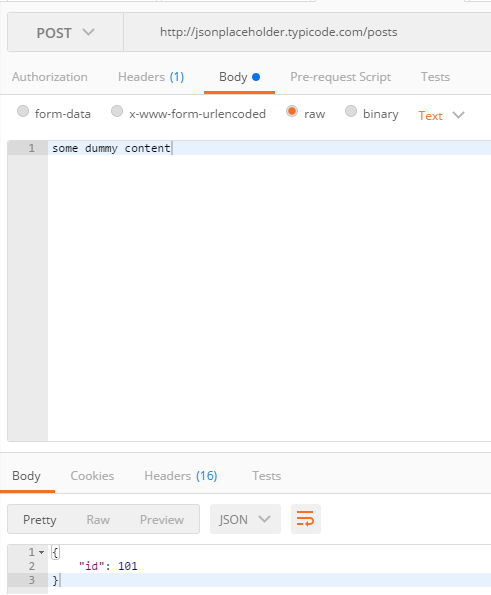
Figure 1 – Output of the HTTP POST request using Postman.
Finally, to get the content of the answer to our request in MicroPython, we just need to access the text property of the Response object. Since the answer is of type JSON, we can also retrieve it as a dictionary object with the parsed content using the json function of the Response object, which uses the ujson library in its implementation.
1 print(response.text)
2 print(response.json())
You can check bellow in figure 2 the result of all the commands shown in this tutorial. As can be seen, we can access both the raw response in a string format or in a parsed JSON object.
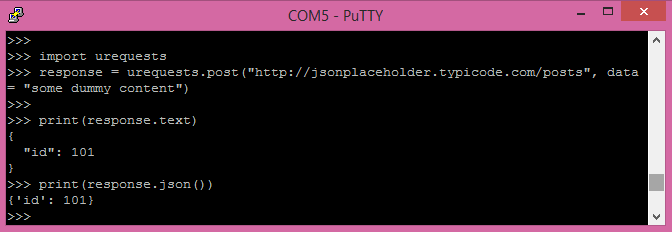
Figure 2 – Result of the HTTP POST request using MicroPython.
NOTE: This article is written by Nuno Santos who is an kindly Electronics and Computers Engineer. live in Lisbon, Portugal. you could check the original article here.
He had written many useful tutorials and projects about ESP32, ESP8266, If you are interested, you could check his blog to know more.