My company car that I normally drive tends to have a "small" problems from time to time, the speedometer fall to 0 Km/h when driving (after some time it resumes again).
Normally this is not a big issue since if you know how to drive a car, you are not, I hope, always looking to the speedometer. You now more or less the speed that you are driving.
The problem present it self when you need to decrease speed to the road limit that you are entering and you notice that "the speedometer is down".
This presented like a good opportunity to build a new project, "The GPS Speedometer".
Of course the ideal solution would be, really repair the car or use a normal GPS or use an app with this function but what would be the fun in this :)
Hardware components:
Microcontroller
I selected the DFRobot Dreamer Nano V4.1 because it has a usb plug that I can use for power and a compatible breadboard pinout.
Check DFRobot Dreamer Nano wiki page for more info regarding this microcontroller
GPS
I'm using the UBX-G7020-KT, that comes with an integrated antenna and allows to change the refress rate until 10Hz(for this project this featuring can came in hand).
On the DFRobot GPS Module wiki page you will find more info regarding it.
Display
I wanted to have a good display without "blowing" the budget, my choose was
the OLED 2828 Display Module. Check again the oled Display module wiki page for some more info.
Power
The power for the system will be provided by the car cigarette lighter socket.
Casing
This time I when for designing a casing and 3D printing it. After some attempts, in the end I was hopping to get something like this.
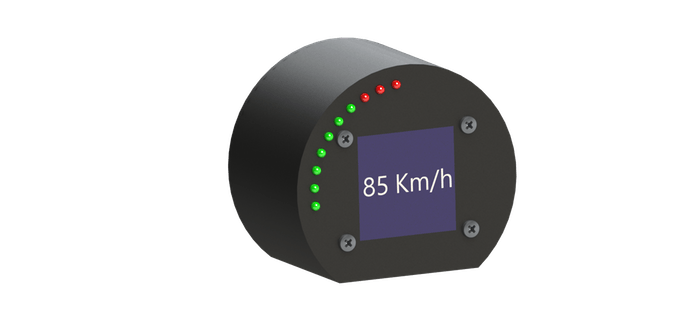
Components
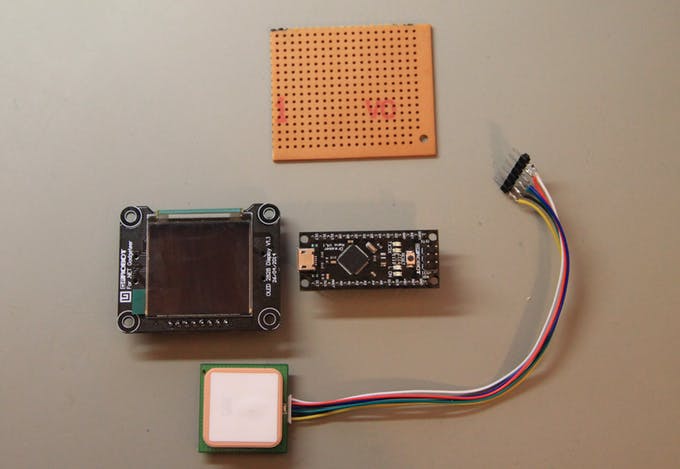
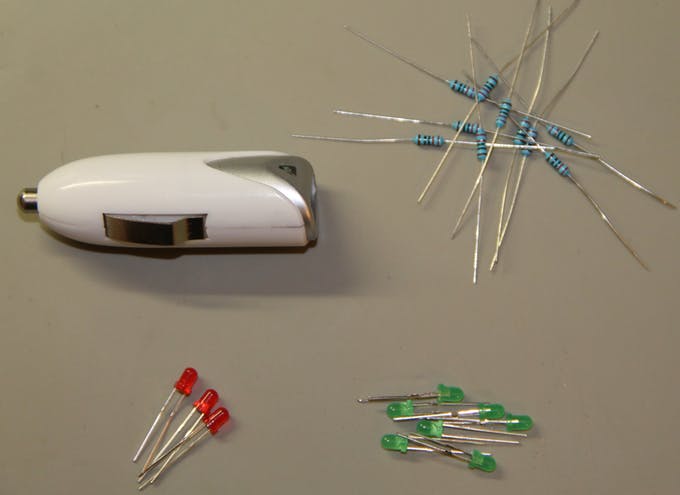
Connect the components
The design with the LED's was not my first choice. So initially I designed this schematic for the circuit.
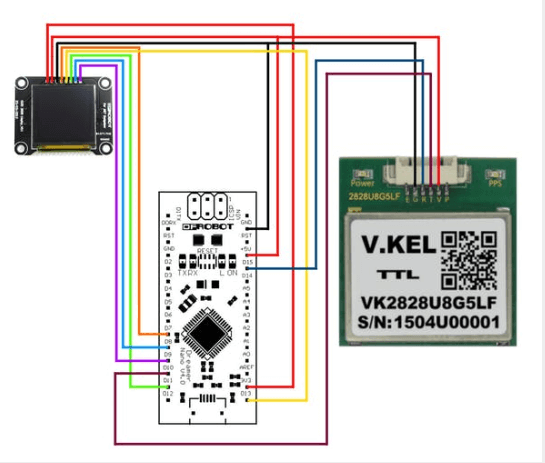
But in the end the final result was this.
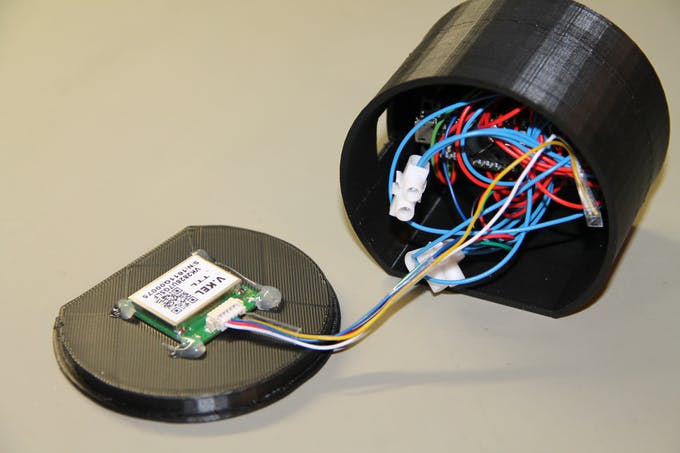
I forgot to take some pictures of the assembly process, so what I can say is that everything is assembled in the prefboard, in one side is the oled display and in the other the microcontroller and connections. In order to make this easy leave the oled display for last since some of the connections will be done on its back.
Code
In order to run the code you will need to have installed the following library 's in your Arduino Library Folder.
U8glib - For the oled display.
TinyGps++ - For the GPS.
The code is "printing" the speed, course, number of satellites, latitude and longitude.
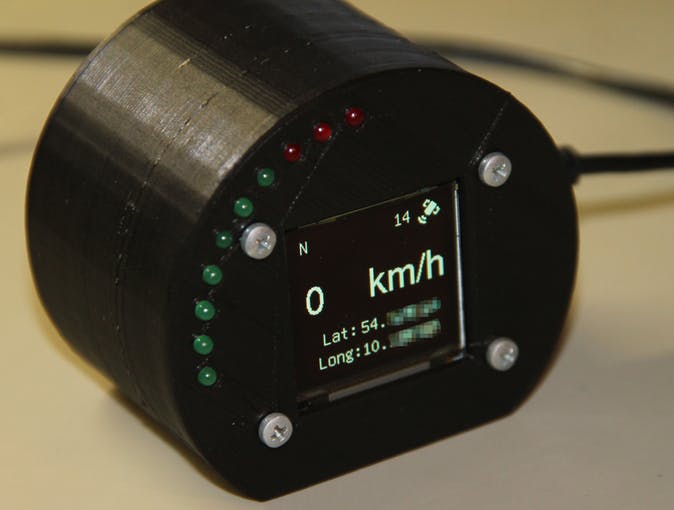
But it is possible to display much more information, ex.: time, date, distance to point...
Check the full example of the TinyGPS++ library to see all available options that you can have regarding the information retrieved by the GPS.
The other featuring is the LED's bar. I've set it up for a max. of 190Km/h. I live in Germany and some of the highways do not have limits, if not, I would place +/- the maximum road limit available.
Simply change the limit in the "map" function to the one that fits your needs.
Conclusion
I still relatively new in the 3D printing world, so it is natural that my prints do not come perfect :)
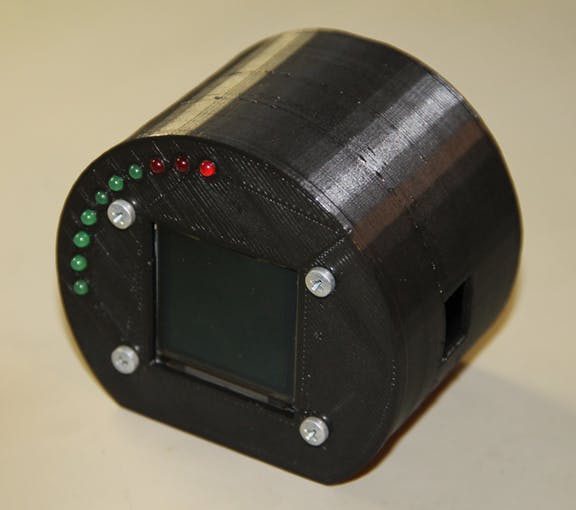
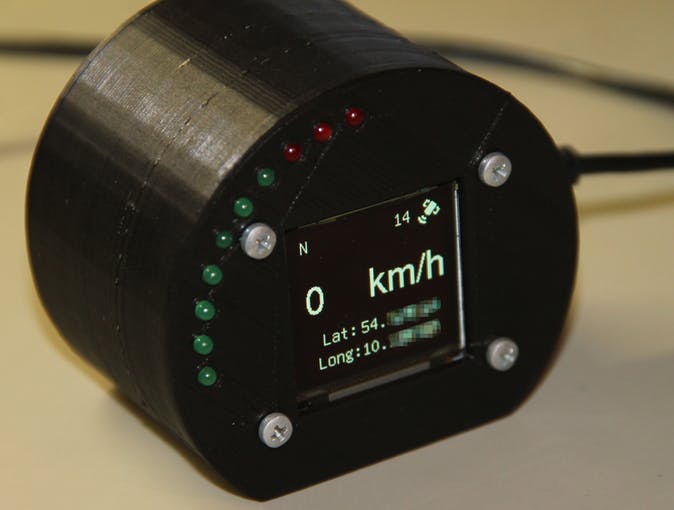
In general I can not complain but I still have a lot to improve on this area.
The back plate currently is not fixing go good as in the beginning, so some more design updates will be needed.
Also I left out the GPS antenna in the back plate, thing that I will not do in the next design.
The course display also did not work so well, but this was only to a little detail. In the future I'm planning to replace with something more useful, ex.: time of arrival to a point (size the majority of my trips are going back and forward).
Fell free to comment or send me a message if you found any mistake or if you have any suggestion/improvement or questions.
"Do not get bored, do something".
Schematic
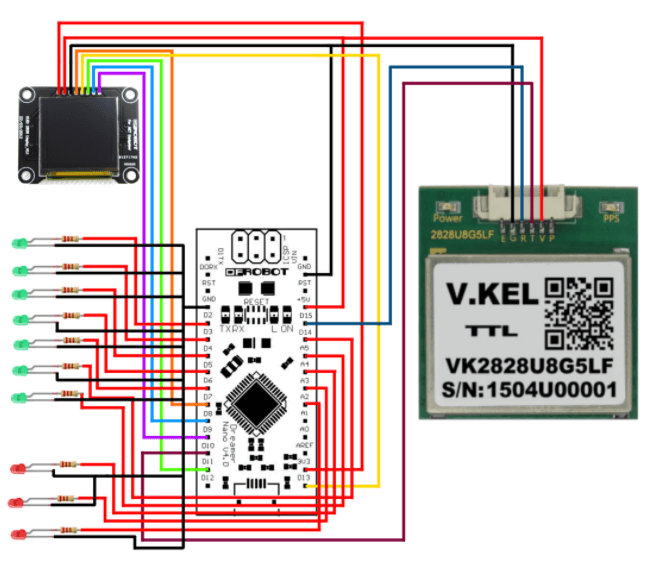
CODE
#include "U8glib.h"
U8GLIB_SSD1351_128X128_332 u8g(13, 11, 8, 9, 7); // Arduino UNO: SW SPI Com: SCK = 13, MOSI = 11, CS = 8, DC = 9, RESET = 7
#define u8g_logo_sat_width 20
#define u8g_logo_sat_height 20
//satellite logo
const unsigned char u8g_logo_sat[] = {
0x00, 0x01, 0x00, 0x80, 0x07, 0x00, 0xc0, 0x06, 0x00, 0x60, 0x30, 0x00,
0x60, 0x78, 0x00, 0xc0, 0xfc, 0x00, 0x00, 0xfe, 0x01, 0x00, 0xff, 0x01,
0x80, 0xff, 0x00, 0xc0, 0x7f, 0x06, 0xc0, 0x3f, 0x06, 0x80, 0x1f, 0x0c,
0x80, 0x4f, 0x06, 0x19, 0xc6, 0x03, 0x1b, 0x80, 0x01, 0x73, 0x00, 0x00,
0x66, 0x00, 0x00, 0x0e, 0x00, 0x00, 0x3c, 0x00, 0x00, 0x70, 0x00, 0x00
};
// The serial connection to the GPS device
#include <SoftwareSerial.h>
static const int RXPin = 10, TXPin = 15;
static const uint32_t GPSBaud = 9600;
SoftwareSerial ss(RXPin, TXPin);
//GPS Library
#include <TinyGPS++.h>
TinyGPSPlus gps;
//Program variables
double Lat;
double Long;
//int day, month, year;
//int hour, minute, second;
int num_sat, gps_speed;
String heading;
const int ledCount = 10; // the number of LEDs in the bar graph
int ledPins[] = {
2, 3, 4, 5, 6, 14, A5, A4, A3, A2
}; // an array of pin numbers to which LEDs are attached
void setup() {
ss.begin(GPSBaud);
// assign default color value
if ( u8g.getMode() == U8G_MODE_R3G3B2 ) {
u8g.setColorIndex(255); // white
}
//Define ledpins as OUTPUT's
for (int thisLed = 0; thisLed < ledCount; thisLed++) {
pinMode(ledPins[thisLed], OUTPUT);
}
}
void loop() {
Get_GPS(); //Get GPS data
Led_Bar();//Adjust the LED bar
//Display info in the OLED
u8g.firstPage();
do {
print_speed();
} while ( u8g.nextPage() );
}
void print_speed() {
u8g.setFont(u8g_font_helvR24r);
u8g.setPrintPos(2, 70);
u8g.print(gps_speed , DEC);
u8g.setPrintPos(60, 70);
u8g.print("km/h");
u8g.setFont(u8g_font_unifont);
u8g.setPrintPos(85, 15);
u8g.print( num_sat, 5);
u8g.setFont(u8g_font_unifont);
u8g.setPrintPos(15, 100);
u8g.print("Lat:");
u8g.setPrintPos(50, 100);
u8g.print( Lat, 6);
u8g.setPrintPos(10, 120);
u8g.print("Long:");
u8g.setPrintPos(50, 120);
u8g.print( Long, 6);
u8g.setFont(u8g_font_unifont);
u8g.setPrintPos(0, 15);
u8g.print( heading);
u8g.drawXBM(108, 0, u8g_logo_sat_width, u8g_logo_sat_height, u8g_logo_sat);
}
// This custom version of delay() ensures that the gps object
// is being "fed".
static void smartDelay(unsigned long ms)
{
unsigned long start = millis();
do
{
while (ss.available())
gps.encode(ss.read());
} while (millis() - start < ms);
}
void Get_GPS()
{
num_sat = gps.satellites.value();
if (gps.location.isValid() == 1) {
Lat = gps.location.lat();
Long = gps.location.lng();
gps_speed = gps.speed.kmph();
heading = gps.cardinal(gps.course.value());
}
/*
if (gps.date.isValid())
{
day = gps.date.day();
month = gps.date.month();
year = gps.date.year();
}
if (gps.time.isValid())
{
hour = gps.time.hour();
minute = gps.time.minute();
second = gps.time.second();
}
*/
smartDelay(1000);
if (millis() > 5000 && gps.charsProcessed() < 10)
{
// Serial.println(F("No GPS detected: check wiring."));
}
}
void Led_Bar()
{
int ledLevel = map(gps_speed, 0, 190, 0, ledCount);
for (int thisLed = 0; thisLed < ledCount; thisLed++) {
// if the array element's index is less than ledLevel,
// turn the pin for this element on:
if (thisLed < ledLevel) {
digitalWrite(ledPins[thisLed], HIGH);
} else { // turn off all pins higher than the ledLevel:
digitalWrite(ledPins[thisLed], LOW);
}
}
}
This project was made by Hugo Gomes, the original project link is here.