The objective of this ESP32 / ESP8266 MicroPython Tutorial is to explain how to use dictionaries in MicroPython. This tutorial was tested both on the ESP32 and on the ESP8266. The tests on the ESP32 were performed using a DFRobot’s ESP-WROOM-32 device integrated in a ESP32 FireBeetle board.
Introduction
The objective of this ESP32 / ESP8266 MicroPython Tutorial is to explain how to use dictionaries in MicroPython. Naturally, the functionalities that we will see here also apply to Python, since dictionaries are one of its basic data structures.
Please note that this should be an introductory tutorial about MicroPython dictionaries. Thus, we will not be covering the most advanced functionalities.
The tests were performed using uPyCraft, a MicroPython IDE. The easiest way to follow the tutorial is sending the commands in the MicroPython command line. You can check how to use it on uPyCraft in this previous post.
The tests were performed on both the ESP32 and the ESP8266. The tests on the ESP32 were performed using a DFRobot’s ESP-WROOM-32 device integrated in a ESP32 FireBeetle board. The pictures shown through the tutorial are from the tests on the ESP32.
Creating dictionaries
The syntax to create a dictionary is as simple as enclosing the sequence of key value pairs in curly brackets, separating each key value pair with comas. The separation of the key and the value is done with a colon.
1 Dictionary = {key1:value1, key2:value2, ...}
So bellow we show the declaration of a dictionary where both the keys and the values are strings.
testDictionary = {"key1": "value1", "key2": "value2"}
print(testDictionary)
A particular case is declaring an empty dictionary, where we simply use the curly brackets with nothing inside.
emptyDictionary = {}
print(emptyDictionary)
You can check bellow at figure 1 the expected result from running these commands.

Figure 1 – Output of the dictionary creation commands.
Note that although we have used strings as keys, we can use any type that is hashable and supports equality comparison [1]. You can read more about this subject here. On the other hand, the values of a dictionary can be of any type [2].
Accessing the elements
In order to access elements in a dictionary, we simply use square brackets and put inside the key to which we want to obtain the value. Note that this syntax is similar to the one used in lists, shown here, although now our keys can be different from numbers.
Note that in the example bellow we create a dictionary with different types of objects, more precisely an integer, a string and a list. This is valid, as we will see upon running the code.
testDictionary = {"key1": 1, "key2": "value2", "key3" : [1,2,3]}
print(testDictionary["key1"])
print(testDictionary["key2"])
print(testDictionary["key3"])
Naturally if we try to access a key that is not present in the dictionary, we will obtain an error.
print(testDictionary["nonExistingKey"])
You can check bellow at figure 2 the output of these commands. Note the error thrown when the key doesn’t exist.

Figure 2 – Accessing the elements of the dictionary.
Another way of accessing an element is by calling the get method on the dictionary object and pass as input the key. This returns the value for the key, if it exists. If the key doesn’t exist, this call will return a default value, which can be optionally passed as second parameter of the get method. If this default value is not specified, then the value none is returned if the key is not found.
myDictionary = {"key1": "value1", "key2":"value2", "key3": "value3"}
val = myDictionary.get("key1")
print(val)
val = myDictionary.get("nonExistingKey")
print(val)
val = myDictionary.get("nonExistingKey", "the item was not found")
print(val)
You can check the result of the invocation of the get method bellow, in figure 3. Note that if the key doesn’t exist and we don’t pass an additional value to the method, it will return None, as we have said before. On the other hand, if we want, we can pass a value that will be returned if the item is not found, which was the “the item was not found” string, which is now returned instead of None.

Figure 3 – Use of the get method to obtain dictionary values.
Note that we can also check if a key exists in a dictionary by using the in operator, as can be seen below. Nonetheless, it can’t be used to check if a value exists in the dictionary [3].
myDictionary = {"key1": "value1", "key2":"value2", "key3": "value3"}
"key1" in myDictionary
"value1" in myDictionary
You can confirm in figure 4 the expected result of applying the in operator to both a key and a value of the dictionary.

Figure 4 – Applying the in operator to dictionaries.
To obtain all the keys of a dictionary, we can call the keys method. It will return an object with the keys of the dictionary. Similarly, we can get an object with all the dictionary values with a call to the values method. For an object of tuples composed by the key value pairs of the dictionary, we simply call the items method.
Note that in the 3 cases we refer to objects and not lists because these methods actually return an object of type dict_view, which provide a dynamic view of the dictionary that reflects its changes [4]. We will confirm that this is indeed the type returned by using the type function, passing as input the objects returned by these methods.
However, transforming these objects in Python lists is as easy as calling the list function, passing as input the mentioned objects.
myDictionary = {"key1": "value1", "key2":"value2", "key3": "value3"}
keys = myDictionary.keys()
print(type(keys))
print(list(keys))
values = myDictionary.values()
print(type(values))
print(list(values))
items = myDictionary.items()
print(type(items))
print(list(items))
To get the number of elements in the dictionary, we simply call the len function and pass as input the dictionary.
len(myDictionary)
You can check below at figure 5 the results of these commands.
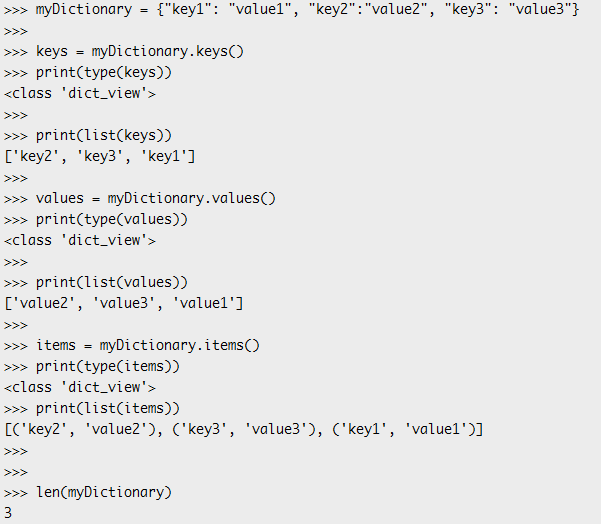
Figure 5 – Output of the previous dictionary methods calls.
Updating and adding elements
Updating and adding elements to the dictionary is equal. Basically, we use square brackets enclosing the key for the value we want to add / modify and assign it a value. If that key doesn’t exist, then the key value pair is added to the dictionary. If it exists, then the value is updated.
emptyDictionary = {}
emptyDictionary["key1"] = "value1"
print(emptyDictionary)
emptyDictionary["key1"] = "updatedValue1"
print(emptyDictionary)
If we want to add the key value pairs, of one dictionary to another, we call the update method on one of them and pass as input the other.
dictionaryToAdd = {"key2":"value2"}
emptyDictionary.update(dictionaryToAdd)
print(emptyDictionary)
You can confirm the expected result of these commands at figure 6, shown below.

Figure 6 – Adding elements and updating a MicroPython dictionary.
Now that we know how to update a dictionary, we will confirm the statement of the previous section which indicates that the dict_view objects should maintain an updated view of the dictionary status. To test it, we will create a dictionary, call the keys method and print the result, and then add another key to the dictionary and print the previously obtained object again.
myDictionary = {"key1": "value1", "key2":"value2", "key3": "value3"}
keys = myDictionary.keys()
print(keys)
myDictionary["newKey"] = "newValue"
print(keys)
As shown in figure 7, the dict_view object gets updated with the new key added to the dictionary.

Figure 7 – Testing of the dict_view object behavior upon an update on the dictionary.
Deleting elements
To delete an element from the dictionary, we simply use the del operator and access the element we want to delete by its key. If we want to delete a whole dictionary, we can apply the del operator to the dictionary (without accessing a specific element).
myDictionary = {"key1": "value1", "key2":"value2", "key3": "value3"}
del myDictionary["key1"]
print(myDictionary)
del myDictionary
print(myDictionary)
To delete all elements of the dictionary, without deleting it , we can call the clear method. This will delete all key value pairs and make our dictionary an empty one.
myDictionary = {"key1": "value1", "key2":"value2", "key3": "value3"}
myDictionary.clear()
print(myDictionary)
Figure 8 shows the previous different dictionary deleting options.
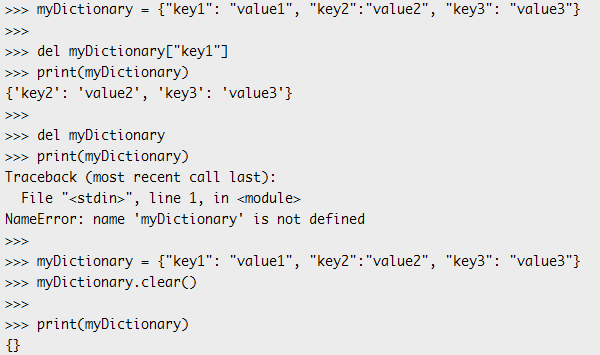
Figure 8 – Dictionary deletion functionalities.
NOTE: This article is written by Nuno Santos who is an kindly Electronics and Computers Engineer. live in Lisbon, Portugal. you could check the original article here.
He had written many useful tutorials and projects about ESP32, ESP8266, If you are interested, you could check his blog to know more.