The objective of this ESP32 / ESP8266 MicroPython Tutorial is to explain how to use the split method to separate strings in sub-strings accordingly to a given separator string. This tutorial was tested both on the ESP32 and on the ESP8266. The tests on the ESP32 were performed using a DFRobot’s ESP32 Module device integrated in a ESP32 development board.
Introduction
The objective of this ESP32 / ESP8266 MicroPython Tutorial is to explain how to use the split method to separate strings in sub-strings accordingly to a given separator string. Although this is very simple to perform in MicroPython, it is very useful for processing textual data.
This tutorial was tested both on the ESP32 and on the ESP8266. The tests on the ESP32 were performed using a DFRobot’s ESP32 Module device integrated in a ESP32 development board.The MicroPython IDE used for the tests was uPyCraft. You can check how to use it on uPyCraft in this previous post.
The split method
Splitting a string in multiple sub-strings is achieved by calling the split method of the string class. This method is called on the string object we want to divide and receives as input another string that corresponds to the separator between each sub-string [1].
The separator may be composed of multiple characters [1] and if not specified it defaults to an empty space [2].
As output, the split method returns a list with the sub-strings found. The separator string is not included in the results.Additionally, the split method supports a delimiter parameter which indicates how many splits should be done at most [1]. If this parameter is not specified, then no limit is applied.
The code
We will start by declaring a very simple string of multiple words separated by empty spaces and test calling the split method without arguments. As told in the previous section, this means that it will assume an empty space (” “) as separator character and no limit to the number of splits performed.
myString = "I will go to the cinema."
print(myString.split())
As can be seen in figure 1, the string gets split by the empty space character and a list with all the sub-strings is returned, without the separator character.

Figure 1 – Output of applying the split method without input arguments.
Now we will test passing as input of the split method a separator string. So, we will declare a string with some words separated by a “|” character, and use this character as input of the split method.myString = "one|two|three"
print(myString.split("|"))
Figure 2 shows the output of these commands. As can be seen, the call to the split method returns the list of sub-strings which were separated by the “|” character.
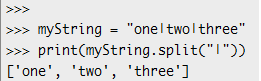
Figure 2 – Applying the split method to a string with words separated by “|”.
If additionally we pass the maximum number of splits argument to the split method, then a different number of sub-strings will be returned.
myString = "one|two|three"
print(myString.split("|",0))
print(myString.split("|",1))
print(myString.split("|",2))
print(myString.split("|",3))
Figure 3 shows the output of adding this parameter. If we specify a maximum of 0 splits, then it means the original string will be returned. If we specify a number greater than the maximum splits that can be applied to the string, then only the sub-strings detected will be returned.
Figure 3 – Limiting the maximum number of splits.
If we use a separator string that is not found on the string to split, then the returned list will have only one element, corresponding to the whole original string.
myString = "one|two|three"
print(myString.split("#"))
This behavior can be confirmed at figure 4.
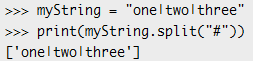
Figure 4 – Output of the split method when no separator character is found.
To finalize, we will confirm that we can use bigger strings as separators, as can be seen in the code below.
myString = "one|separator|two|separator|three"
print(myString.split("|separator|"))
As shown in figure 5, we still get the correct result when using a bigger string as separator.

Figure 5 – String split with a separator greater than one character.
NOTE: This article is written by Nuno Santos who is an kindly Electronics and Computers Engineer. live in Lisbon, Portugal. you could check the original article here.
He had written many useful tutorials and projects about ESP32, ESP8266, If you are interested, you could check his blog to know more.