The objective of this esp32 tutorial is to explain how to use the JavaScript array every method to check if all the elements of a given array pass a certain condition. This will be tested on Espruino running on the ESP32. The tests were performed using a DFRobot’s ESP32 module device integrated in a ESP32 FireBeetle board.
Introduction
The objective of this post is to explain how to use the JavaScript array every method to check if all the elements of a given array pass a certain condition. This will be tested on Espruino running on the ESP32.
The every method is called on an array and receives as input a user defined function. This function is executed for each element of the array and is responsible for implementing the condition we want to check.
The every method will return true if, after applying the provided function to each element of the array, all function calls returned true. Otherwise, the every method returns false.
Regarding the user defined function, it takes three arguments. The first argument is the current element of the array, the second is the index of the current element of the array and the third is the array the every method was called upon.
Note that the two last arguments can be omitted from the function if they are not needed.
The every method will be responsible for passing the previously mentioned arguments to our defined function and thus we will only need to worry about applying the condition.
The tests below were performed using a DFRobot’s ESP32 module device integrated in a ESP32 development board.
The code
We will start our code by declaring our validation function, where we will define the condition to be checked for each element of the array.
We will define a simple function that checks if the current element is greater than 10. If it is, the function returns true. Otherwise, it returns false.
Thus, by supplying this function as input of the every method, that method will return true if all the elements of the array are greater than 10 and false otherwise.
Since we only need to check the current value, we will only specify an input argument for our function.
function checkGreaterThanTen(currentValue){
return currentValue > 10;
}
After defining our function, we will declare two arrays of numbers, one of them meeting the specified criteria for all the elements and the other not.
So, the first one, which we will call arrayTrue, will have all the elements greater than 10 and thus all the elements meet our function’s criteria. The other one, which we will call arrayFalse, will have elements lesser than 10, therefore failing the function’s criteria.
var arrayTrue = [14, 15, 20, 27];
var arrayFalse = [1, 2, 20, 22];
Finally, we will call the every method on each array, passing as input our defined function, and then print the results. You can check the full code below, which already contains these calls.
function checkGreaterThanTen(currentValue){
return currentValue > 10;
}
var arrayTrue = [14, 15, 20, 27];
var arrayFalse = [1, 2, 20, 22];
console.log(arrayTrue.every(checkGreaterThanTen));
console.log(arrayFalse.every(checkGreaterThanTen));<span data-mce-type="bookmark" id="mce_SELREST_start" data-mce-style="overflow:hidden;line-height:0" style="overflow:hidden;line-height:0" ></span>
Testing the code
To test the code simply upload and run the previous script on your ESP32. The easiest way to do it is by using the Espruino IDE.
You should get an output similar to figure 1, which shows that calling the every method on the first array returns true and on the second returns false. This is coherent with the function that we implemented, which checks if the values are greater than 10.
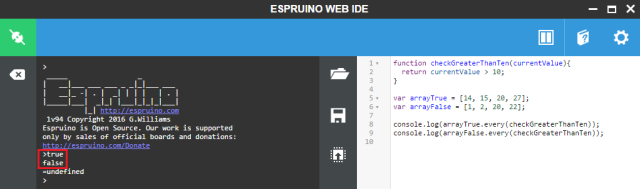
Figure 1 – Output of the program.