The objective of this esp32 tutorial is to explain how to use JavaScript array map method to apply a transformation to a given array, on Espruino running on the ESP32. The tests were performed using a DFRobot’s ESP32 module device integrated in a ESP32 development board.
Introduction
The objective of this post is to explain how to use JavaScript array map method to apply a transformation to a given array. This will be tested on Espruino running on a ESP32.
If you prefer, you can check the video version of this tutorial at my YouTube channel.
The map method
The array map method is a very powerful functionality from JavaScript that allows us to obtain a new array with the results of applying a certain function (defined by us) to every element of the calling array [1].
The mapping function accepts 3 arguments. The first one will correspond to the current element of the array being processed, the second one to the index of the current element being processed, and the third one to the array in which the map method was called [1].
Note however that since we are using JavaScript, we don’t need to specify all the arguments on the mapping function and it will work fine. For example, if we don’t need to access the current index and the whole original array, we can simply define a mapping function with only one argument, to which the current value being processed will be passed.
Naturally, for our mapping function to be useful, this first argument (the current element) will most likely be always needed.
In our tutorial, we will use the map functionality to get all the values of an array incremented by one, in a new array.
The code
We start our code by declaring an array variable with some numbers. This will our original array, to which we will apply the mapping.
var array = [4,5,6,7,8];
Next we need to define the mapping function that will be applied to our array, which we will call mapArray. Note that you can name it however you want. This function will receive two arguments.
As mentioned in the previous section, the first argument will correspond to the current value of the array, to which the map function will be applied. The second one corresponds to the current index of the array, to which the value was passed to our function.
function mapArray(value, index){
//Mapping function implementation
}
The implementation of our function will be very simple. First, we will print the index argument, so we can confirm that the mapping function is indeed applied to each element of the array.
After that, we will simply return the value of the current element incremented by one. This element was passed in the value argument. The full mapping function is shown below.
function mapArray(value, index){
console.log(index);
return value+1;
}
Now that we have our original array and the mapping function, we simply call the map method on our array and pass as input the previously defined mapping function.
Since this call will return the new array after the mapping is applied, we will store the result in a variable.
var newArray = array.map(mapArray);
To finalize our code, we will print both the new array and the original one. Note that the original array should not have been modified. The final source code can be seen below.
var array = [4,5,6,7,8];
function mapArray(value, index){
console.log(index);
return value+1;
}
var newArray = array.map(mapArray);
console.log("New array");
console.log(newArray);
console.log("Original array");
console.log(array);
Testing the code
To test the code, simply upload the previous script to your ESP32 using the Espruino IDE. Upon execution, you should get an output similar to figure 1.
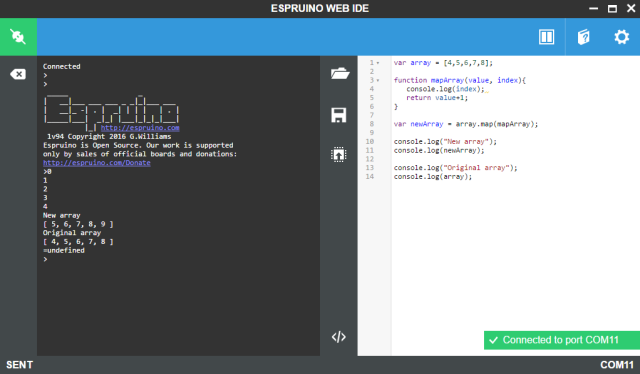
Figure 1 – Output of the array map program.
Note that, as expected, each index of the array is printed by our mapArray function. Next, the new array with each position incremented is correctly printed and the original array is not changed.