Fire alarms can be very useful in our kitchens. In this session we will make our own simple version that can detect flames.
COMPONENTS
HARDWARE CONNECTIONS
Connect the Digital Buzzer Module to digital pin 8 on the Arduino
Connect the Flame Sensor to analog pin 0 on the Arduino
Review the diagram below for reference:
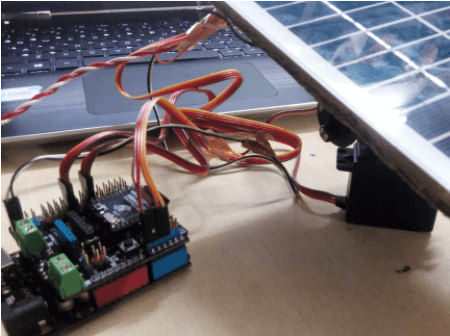
Be sure that your power, ground and signal connections are correct or you risk damaging your components.
When you have connected the components and checked your connections, plug the Arduino in to your PC with the USB cable so you can upload a program.
CODE INPUT
Sample Code 8-1:
// Item Eight- Flame Alarm
float sinVal;
int toneVal;
void setup(){
pinMode(8, OUTPUT); //Set up the buzzer’s pins
Serial.begin(9600); // set the baud rate to be 9600 bps
}
void loop(){
int sensorValue = analogRead(0);// The flame sensor is connected to analog pin 0.
Serial.println(sensorValue);
delay(1);
if(sensorValue < 1023){ // If the sensor value is less than 1023, flame is detected and we sound the alarm
for(int x=0; x<180; x++){
// transfer the sin function to radian
sinVal = (sin(x*(3.1412/180)));
// get the sound frequency with the sin function
toneVal = 2000+(int(sinVal*1000));
//Give a tone to pin 8
tone(8, toneVal);
delay(2);
}
} else { // If the sensor value is greater than 1023, there is no flame and we turn the buzzer off
noTone(8); // turn the buzzer off
}
}
Use this sample code to implement the behavior we want.
You can copy and paste it in to the Arduino IDE, but if you want to develop you skills we recommend typing it out.
When you have finished, click “Verify” to check the code for syntax errors. If the code verifies successfully, you can upload it to your Arduino.
Use a flame from a match or a lighter near the flame sensor. Check if the buzzer sounds.
CODE ANALYSIS
In this code, two variables have been defined:
float sinVal;
int toneVal;
“sinVal” is used to generate a sin value. We will use a sinusoidal wave to create the sound pattern of the alarm. The “sinVal” value will generate the “toneVal” which is an alarm tone that varies in pitch.
To avoid negative numbers being generated by the sin() function, we use angles between 0 and 180 degrees. We will iterate them in a “for” loop:
for(int x=0; x<180; x++){}
The function sin() uses radians. We need to convert our angles in degrees into radians by multiplying 3.1412/180.
sinVal = (sin(x*(3.1412/180)));
Then we use the resulting value to generate the sound frequency of the buzzer.
toneVal = 2000+(int(sinVal*1000));
Let’s discuss floating point and integer values.
A floating point value may contain decimals. For example: 120.11
An integer has no decimals. For example: 5
“sinVal” is a floating-point variable.
To transform the floating point variable in to an integer we use the following line of code:
int(sinVal*1000)
We multiply sinVal by 1000, transform it into an integer, and then add 2000 to it to get the value for toneVal. Now toneVal is a suitable sound frequency.
We then use this value in the function of “tone()”. The buzzer is able to generate sound using this value.
tone(8, toneVal);
Three functions related to tone are as follows:
tone(pin,frequency)
Pin refers to the digital pin connected to the buzzer. Frequency is a value we measure in Hz.
tone(pin,frequency,duration)
Duration is a parameter we measure in milliseconds. It determines the duration of the buzz. In the first function, there is no duration and the buzz will continue indefinitely.
noTone(pin)
noTone(pin) is used to end the buzz on the designated pin.
EXERCISE
Combine the buzzer with a thermo sensor and a Digital Piranha LED-R and you can make a mini security alarm. It should make a sound and turn on the LED when it detects someone’s presence.