Arduino Tutorial 8: Vibration Sensor
In this project we are going to use the tilt sensor included in your arduino starter kit. The tilt sensor can detect basic motion and orientation. It contains two contacts and a small metal ball. Once held at a particular orientation, the ball bridges the two contacts and completes the circuit. We have also added an LED to this project. When the sensor detects movement, the LED lis HIGH (on). When no movement is detected the LED is LOW (off).
Component
Resistor 220xRx2
Tilt Switch Sensorx1
Circuit
A tilt sensor behaves much like a push button. You need to add a pulldown resistor to the sensor to ensure the circuit is disconnected when no signal is detected.
You also need to add a current-limiting resistor to the LED.
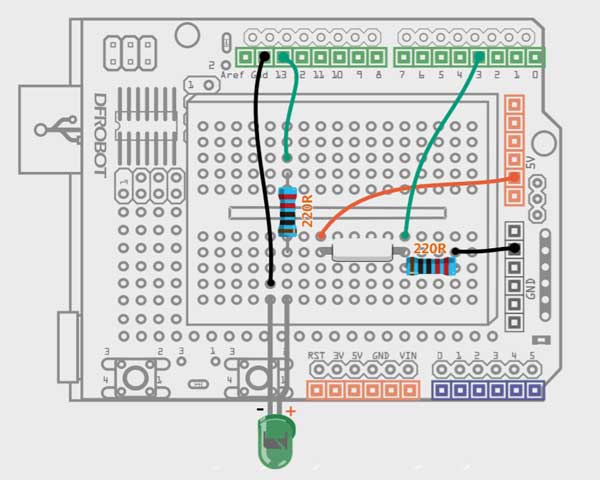
Diagram of the tilt sensor circuit
Code
//project 8 – Vibration sensor
int SensorLED = 13; //define LED digital pin 13
int SensorINPUT = 3; // connect tilt sensor to interrupt 1 in
// digital pin 3
unsigned char state = 0;
void setup() {
pinMode(SensorLED, OUTPUT); //configure LED as output mode
pinMode(SensorINPUT, INPUT); //configure tilt sensor as input mode
//when low voltage changes to high voltage, it triggers interrupt 1 and runs
//the blink function
attachInterrupt(1, blink, RISING);
}
void loop(){
if(state!=0){ // if state is not 0
state = 0; // assign state value 0
digitalWrite(SensorLED,HIGH); // turn on LED
delay(500); // delay for 500ms
}
else{
digitalWrite(SensorLED,LOW); // if not, turn off LED
}
}
void blink(){ // interrupt function blink()
state++; //once trigger the interrupt, the state
} //keeps increment
Expected behaviour:
When we shake the board, the LED is HIGH (on). When we stop shaking, the LED is LOW (off).
Code
In this section we are going to examine the interrupt function that we used in the code. The program works as follows: when there is no interruption to the program, the code keeps running and the LED stays LOW (off). When there is an external event and the tilt sensor is activated, such as someone shaking the board, the program runs the blink() function and state starts incrementing. When the if statement detects that the state is no longer 0, it triggers the LED to be HIGH (on). At the same time, it resets state to 0 and waits for the next interruption. If there is no interruption, the LED is LOW (off).
What is interruption?
Imagine you are watching TV at home and the phone rings. You have to stop watching TV and pick up the phone. After the call has ended, you continue to watch TV. In this case, the call is the interrupt and the ringing of the phone is the condition.
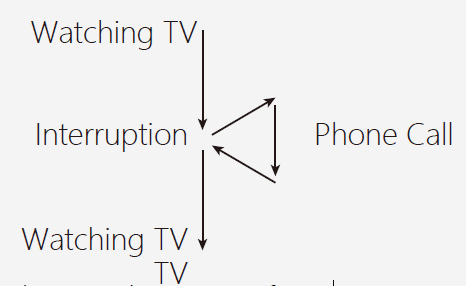
The attachInterrupt function
specifies a named function, or an Interrupt Service Routine (ISR), to call upon when an interrupt occurs. This replaces any previous function that was attached to the interrupt. Most Arduino boards have two external interrupts:
numbers 0 (on digital pin 2) and 1 (on digital pin 3). Different boards have different interrupt pins.
Check the references on arduino.cc
for details: http://arduino.cc/en/
Reference/AttachInterrupt
There are 3 parameters in "attachInterrupt"
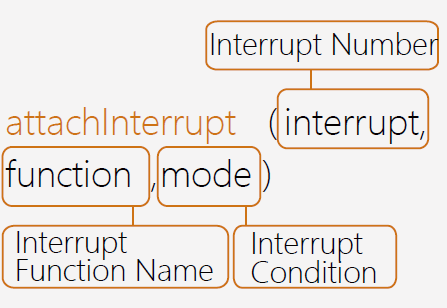
"interrupt":
The number of the interrupt (int), is either number 0 or 1. If it is 0, you must connect the jumper wire to digital pin 2. If it is 1, you must connect the jumper wire to digital
pin 3.
"function" :
- The function is called upon when the interrupt occurs
- The function has no parameters and returns nothing.
- As delay() and millis() both rely on interrupts, they will not work while the function is running.
- The function cannot read values from the serial port. You might lose data connected from serial port.
"mode" :
"mode" defines when the interrupt should be triggered. Four contstants are predefined as valid values:
"LOW" to trigger the interrupt whenever the pin is low,
"CHANGE" to trigger the interrupt whenever the pin changes value
"RISING" to trigger when the pin goes from LOW to HIGH,
"FALLING" for when the pin goes from HIGH to LOW.
Now let’s go back to the program:
attachInterrupt(1, blink,
RISING);
- attachInterrupt(1, because the tilt sensor is connected to digital pin 3.
- Blink is our interrupt function
- RISING , to trigger when the pin goes from LOW to HIGH.
Why have we chosen RISING ? When the tilt sensor does not detect any signal, pin 3 is LOW. When it detects a signal, it connects with 5 volts and this change the pin from LOW to HIGH.
Components
Tilt Sensor
The tilt sensor goes by many different names: ball switch, bead switch, v ibration switch, etc.
Though it has different names, it’s working principles are same. In simple terms, it is a switch made up of a cylinder a small metal ball inside. When the metal ball rolls to either edge of the cylinder, they touch one of the contact pins and the circuit is complete. Examine the diagram for further detail.
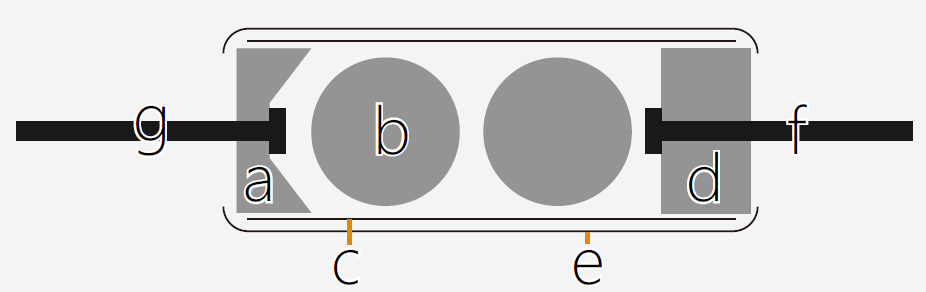
a. Bronze Cover b. Bronze Bead c. Bronze Pipe
d. PC set e. Heat-shrinkable Pipe f . Bronze Conductive Pin g. Phosphor Copper Pinch Cock
Fig. 8-2 Vibration Sensor Diagram