You probably used a remote to turn your TV on an off. A typical remote uses infra-red (IR) light to send information to its host device. In this session we will use the same method to turn an LED on and off.
First, let’s do a warm-up exercise which will help you understand how to use the IR remote control and receiver.
WARM-UP EXPERIMENT
COMPONENTS
HARDWARE CONNECTIONS
Connect the IR Receiver Module V2 to digital pin 10 on the DRFduino.
Use the diagram below for reference:
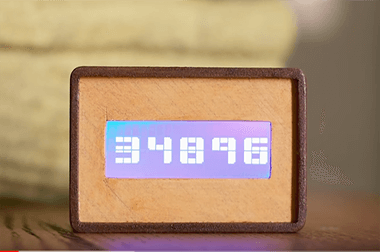
Be sure that your power, ground and signal corrections are correct or you risk damaging your components.
When you have connected the components and checked your connections, plug the Arduino in to your PC with the USB cable so you can upload a program to the DFRduino.
CODE INPUT
You can find the code for this session in the IRremote library under the Lesson12_1 file.
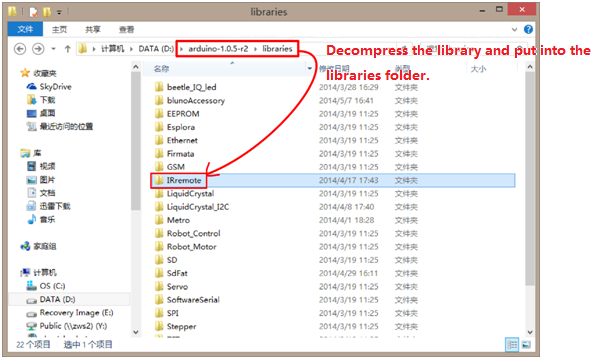
Run the IRrecvDemo code in the examples.
Sample Code 12-1:
//This code comes from IRrecvDemo under examples from the IRremote library
// ITEM TWELVE- IR Receiver
#include <IRremote.h> // include IRremote.h library
int RECV_PIN = 10; //define RECV_PIN (variable) to be 10
IRrecv irrecv(RECV_PIN); // Set RECV_PIN (pin11) as the IR receiver
decode_results results; // define results (variable) to be where results of the IR receiver is located
void setup(){
Serial.begin(9600); //Set the baud rate to be 9600
irrecv.enableIRIn(); //enable IR decoding
}
void loop() {
// if the decoding data has been received; store that data into results
if (irrecv.decode(&results)) {
//Show the data in hexadecimals in the serial monitor
Serial.println(results.value, HEX);
irrecv.resume(); // wait to receive the next group of signals
}
}
Use this sample code to implement the behavior we want.
You can copy and paste it in to the Arduino IDE, but if you want to develop you skills we recommend typing it out.
When you have finished, click “Verify” to check the code for syntax errors. If the code verifies successfully, you can upload it to your DFRrduino.
Open Arduino IDE’s serial Monitor and set its baud rate to be 9600.

Hold the mini remote control against the IR receiver and press one of the buttons. You should see corresponding code on the serial monitor. When you press button “0”, serial port will receive the hexadecimal code FD30CF.

If you keep pressing one button, then “FFFFFFFF” will pop up in the edit box.
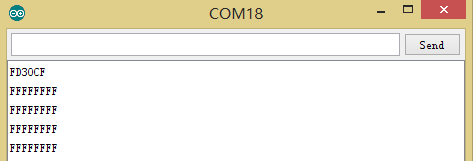
Normally, you will receive a six-digit number that starts with “FD…“. If you do not aim at the IR receiver with remote control in your hands, serial port might receive the wrong code.
The code above has been saved in the IRremote library ready for use.
Now let’s begin the remote controlled LED experiment.
COMPONENTS
HARDWARE CONNECTIONS
Here the additional LED light will be attached to digital pin 10. IR Receiver Module V2 will be attached to digital pin 3.
The connections are similar to the warm up.
Connect the Digital Piranha LED to digital pin 10 on the DFRduino
Connect the IR Receiver Module V2 to digital pin 3 on the DFRduino
Use the diagram below for reference:
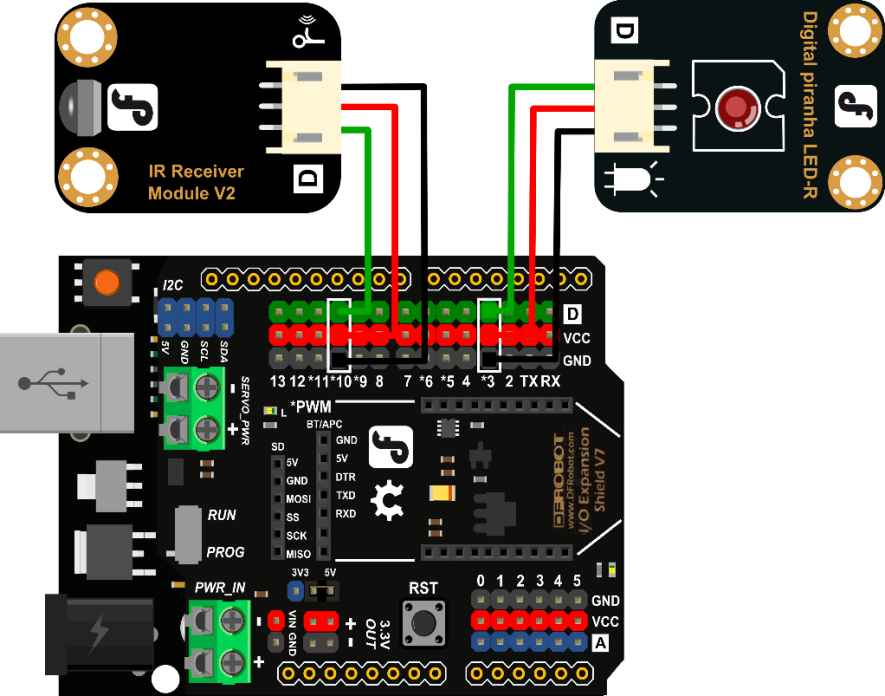
Be sure that your power, ground and signal connections are correct or you risk damaging your components.
When you have connected the components and checked your connections, plug the DFRrduino in to your PC with the USB cable so you can upload a program.
CODE INPUT
Here we suggest you modify code we have just written instead of writing it from scratch.
Sample Code 12-2:
#include <IRremote.h>
int RECV_PIN = 10;
int ledPin = 3; // LED – digital 3
boolean ledState = LOW; //ledstate for storage of LED’s state
IRrecv irrecv(RECV_PIN);
decode_results results;
void setup(){
Serial.begin(9600);
irrecv.enableIRIn();
pinMode(ledPin,OUTPUT); // set LED to be in the output mode
}
void loop() {
if (irrecv.decode(&results)) {
Serial.println(results.value, HEX);
// Once the code for the power button has been received, turn the mode of LED from HIGH to LOW or the other way.
if(results.value == 0xFD00FF){
ledState = !ledState; // reverse it
digitalWrite(ledPin,ledState); //Change the state of LED
}
irrecv.resume();
}
}
Use this sample code to implement the behavior we want.
You can copy and paste it in to the Arduino IDE, but if you want to develop you skills we recommend typing it out.
When you have finished, click “Verify” to check the code for syntax errors. If the code verifies successfully, you can upload it to your DFRrduino.
CODE REVIEW
First we make some declarations regarding the IR sensor.
#include <IRremote.h> //Include IRremote.h library
int RECV_PIN = 10; // define RECV_PIN (variable) to be 10
IRrecv irrecv(RECV_PIN); // Set RECV_PIN (pin11) as the IR receiver
decode_results results; // define results (variable) to be where results of the IR receiver is located
int ledPin = 3; // LED – digital 3
boolean ledState = LOW; // ledstate is used to define LED’s state
Here we’ve defined another variable “ledState” which stores the LED’s state
The function “setup()” will set up the serial port, enable IR decoding and set the mode of digital pins.
The function “loop()”will first check if the Infrared code has been received and save the data collected in results.
if (irrecv.decode(&results))
Once data has been collected, the program will check if the Infrared code has been received.
if(results.value == 0xFD00FF)
Then, it will change the LED’s state.
ledState = !ledState; // reverse
digitalWrite(ledPin,ledState); // Change LED’s state
The exclamation mark “!” means “not equal to”. Therefore, “!ledState” is the opposite of “ledState”. “!” can only be used for boolean values.
Finally, the program will wait for the next group of signals.
irrecv.resume();
If you have any questions, please feel free to comment.
There is also another best selling arduino starter kit for you to choose.