In this session we are going to make a digital dice. When a button is pushed, a random number will be generated. You can use it to play games at home!
COMPONENTS
HARDWARE CONNECTIONS
Connect the Digital Push Button Module to digital pin 2 on the DFRduino UNO
Connect the 8 segment LED Module to the Shiftout Module. Be sure that VCC, ground and signal connections are correct.
Connect the input pins on the shiftout to digital pins on the DFRduino UNO.
Refer to the diagram below:
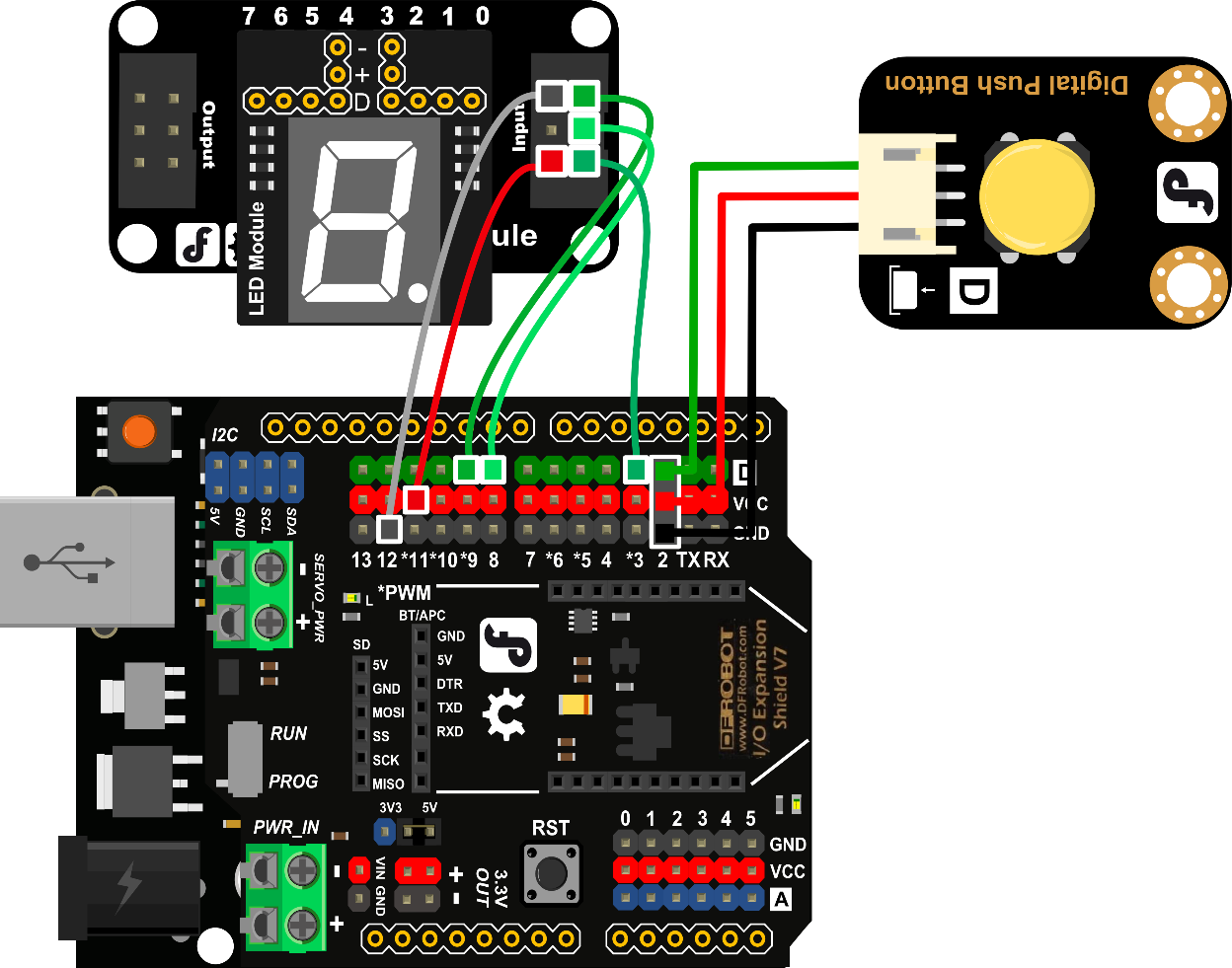
Be sure that your power, ground and signal connections are correct or you risk damaging your components.
When you have connected the components and checked your connections, plug the Arduino in to your PC with the USB cable so you can upload a program.
CODE INPUT
Sample Code 13-1:
// Item Thirteen- Digital Dice
int latchPin = 8; //Digital pin 8 shall be attached to the latch pin of chip 74HC595
int clockPin = 3; //Digital pin 3 shall be attached to the clock pin of chip 74HC595
int dataPin = 9; //Digital pin 9 shall be attached to the data pin of chip
int buttonPin = 2; // The button shall be attached to digital pin 2
// Number 0~9
byte Tab[]={
0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90};
int number;
long randNumber;
void setup() {
pinMode(latchPin, OUTPUT);
pinMode(dataPin, OUTPUT);
pinMode(clockPin, OUTPUT);
randomSeed(analogRead(0)); //set analog pin 0 to be a random seed
}
void loop(){
randNumber = random(10); //result in a random number between 0 and 9
showNumber(randNumber); // show that random number
//Once a button has been pressed, a number will show up
while(digitalRead(buttonPin) == HIGH){
delay(100);
}
}
// This function is for the display of Nixie tube
void showNumber(int number){
digitalWrite(latchPin, LOW);
shiftOut(dataPin, clockPin, MSBFIRST, Tab[number]);
digitalWrite(latchPin, HIGH);
delay(80);
}
A random number between 0 and 9 will show up on the Nixie tube
HARDWARE ANALYSIS
Shiftout Module
The Shiftout Module is a 74HC595 chip. To understand the code, you need a brief idea of how the 74HC595 chip operates. The 74HC595 chip enables serial entry and parallel output.
This figure below shows the difference between serial and parallel operations. Serial operation means digits are sent out one after another, while parallel operation sends out all eight numbers at one time.
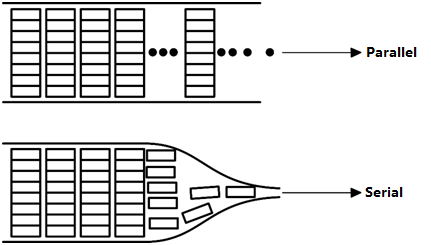
Chip 74HC595 can convert serial data into parallel data and then send it out. When we have to use multiple LED lights and don’t have enough digital pins, chip 74HC595 can solve that problem.
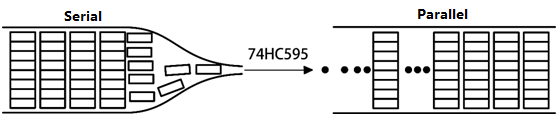
What data do we send? That’s all up to three values: “data”, “latch” and “clock”. Arduino has the function “shiftOut()”, which makes it easier to use Chip 74HC595.
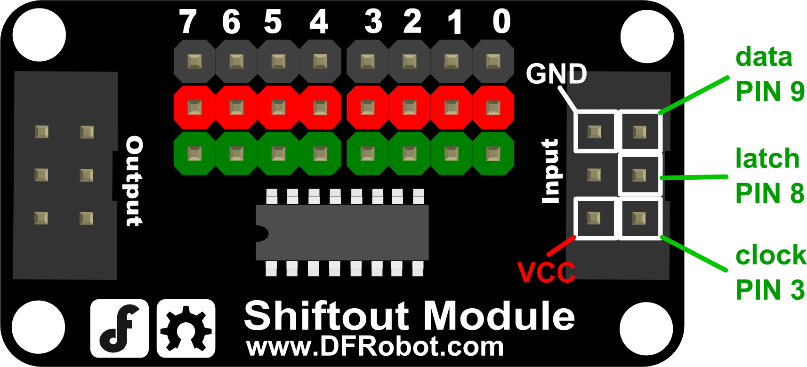
LED Module
The 8 segment LED Module is composed of eight LEDs with one LED for each section. Chip 74HC595 will also send out an eight-digit number. This means that the output of Chip 74HC595 can control the whole LED Module.
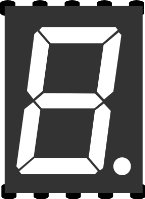
CODE ANALYSIS
Chip 74HC595 makes it possible for a pin to send out 8 signals instead of using eight pins simultaneously for the 8 segment LED.
There are three essential pins “latchPin”, “clockPin” and “dataPin”, so we define them in the beginning.
How to use the function of shiftOut()?
The function of shiftOut() is written in the following form.
shiftOut(dataPin,clockPin,bitOrder,value)
Note:
1. ShiftOut can only be used to send one byte. Therefore if a value is larger than 255, you need to separate the value and do it more than once.
2. dataPin and clockPin should be set as OUTPUT using pinMode() in the setup() function.
REFERENCE:
http://wiki.dfrobot.com.cn/index.php/ShiftOut()
http://arduino.cc/en/Reference/ShiftOut
http://arduino.cc/en/Tutorial/ShiftOut
We can tell from the code that the bitOrder is MSBFIRST. Tab[number] is the data output.
shiftOut(dataPin, clockPin, MSBFIRST, Tab[number]);
Now let’s take a look of Tab[number].
byte Tab[]={
0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90};
These numbers beginning with 0x are in hexadecimal notation. In hexadecimal notation, each digit can be from 0 to 15. We use a~f to represent 10~15.
For better understanding, you can open the calculator on your computer and select the “programmer mode”. Select hexadecimal, type in “c0” and switch to binary, and you will see “11000000”.
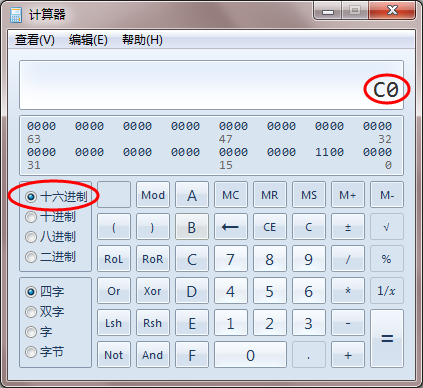
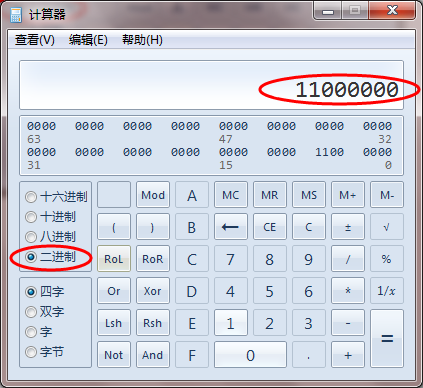
You may still wonder how the numbers relate to the LED.
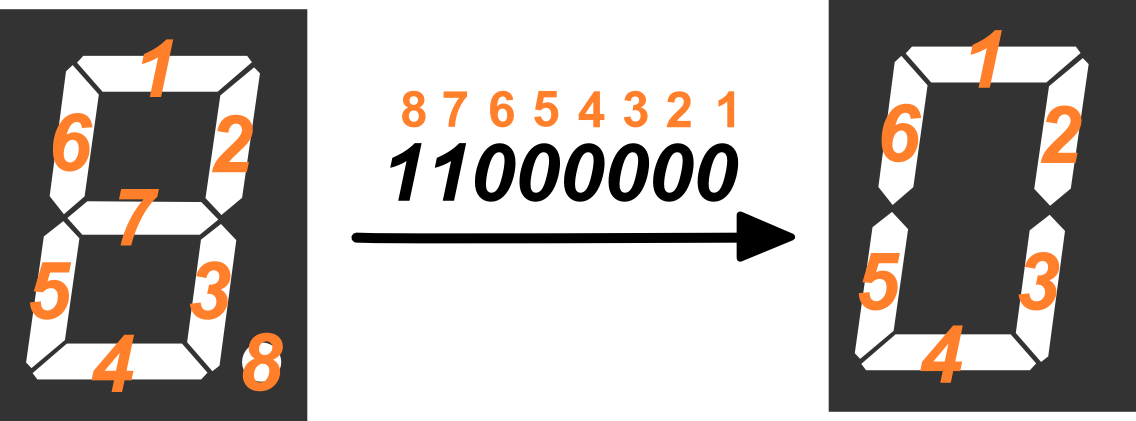
As shown in the diagram above, each binary digit controls one stroke on the LED module. Here “0” means the LED is turned on and “1” means the LED is turned off.
Now that we know how to display 0-9 on the display, we’ll move forward to see how to produce a random number between 0-9. To achieve this, we use the “random()” function.
random(max)
“random()” will generate a random number between 0 and max-1.
random(10);// generate a random number between 0 and 9
randomSeed() is used to generate the Random Seed. Here we’ve attached it to analog pin 0.
randomSeed(analogRead(0));
EXERCISE
You can also combine the LED Module with the IR receiver mentioned in the previous sessions to make a device that displays numbers you press on the IR controller.
We hope these sessions are just the beginning of your robotics journey.
Be creative and have fun playing with your DFRduino and components. If you would like to share your Arduino journey with us, please log in to our forum and tell us your story with Arduino.
You are welcome to the DFRobot maker community!
DFRobot Maker Community: www.dfrobot.com
Thank you and good luck!