This is the fifth tutorial of the ‘How to Build a Robot’ Series. In this tutorial, we will combine the robot platform with the CO2 sensor so that you can detect the CO2 density of a certain area.
Same as the last four tutorials, an Arduino robot kit (Pirate: 4WD Arduino Mobile Robot Kit with Bluetooth 4.0 ) is used here as an example.
Lessons Menu:
Lesson 1: Introduction
Lesson 2: Build a Basic Arduino Robot
Lesson 3: Build a Line Tracking Arduino Robot
Lesson 4: Build an Arduino Robot That Could Avoid Obstacles
Lesson 5: Build an Arduino Robot With Light And Sound Effects
Lesson 6: Build an Arduino Robot That Could Monitor Environment
Lesson 7: Build a Bluetooth-Controlled Arduino Robot
Hardware components
CO2 Gas Sensor for Arduino × 1
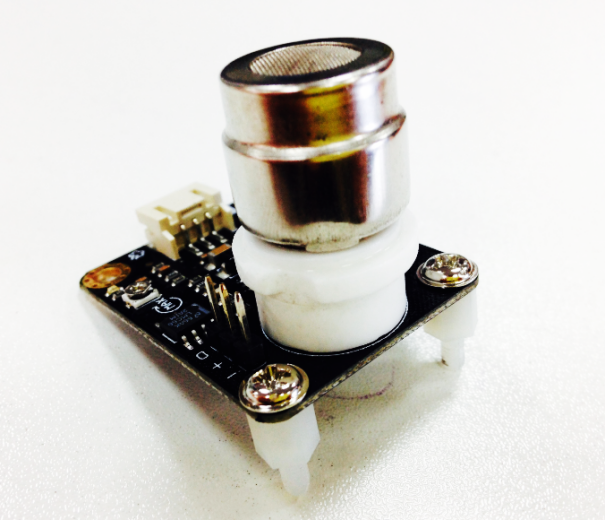
Digital Touch Sensor × 1
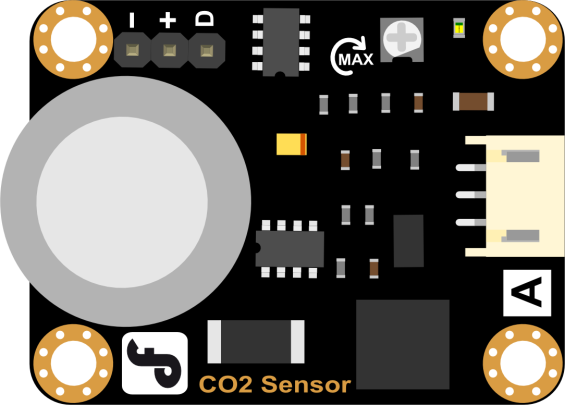
LCD Keypad Shield For Arduino × 1
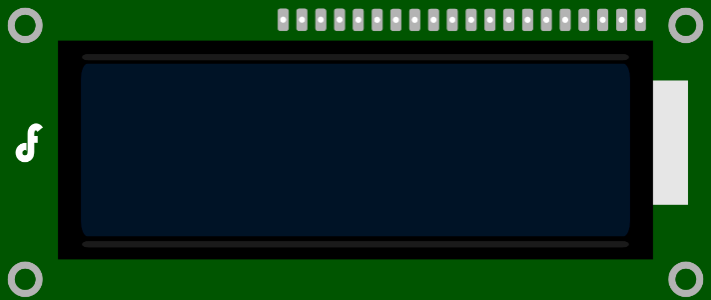
M3*6MM Nylon columns and tie-wraps
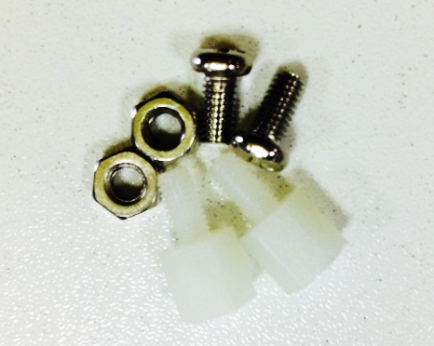
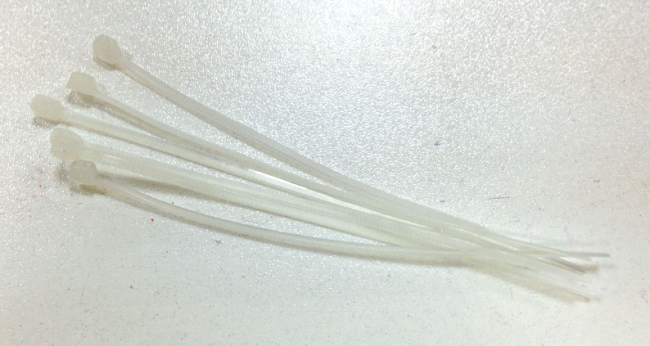
ASSEMBLY INSTRUCTION:
STEP 1: Add the touch sensor
There are two holes on the touch sensor for fixing the Nylon columns.
Fix the Nylon columns. Please do not over-twist those columns.
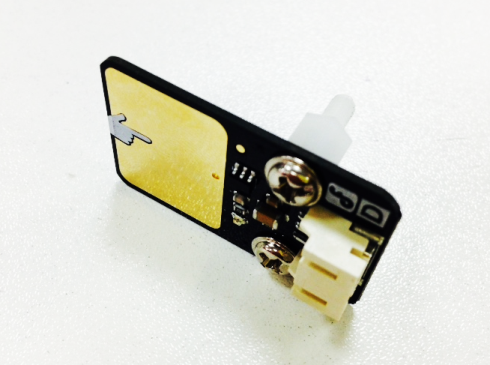
Then attach the touch sensor on the plate.
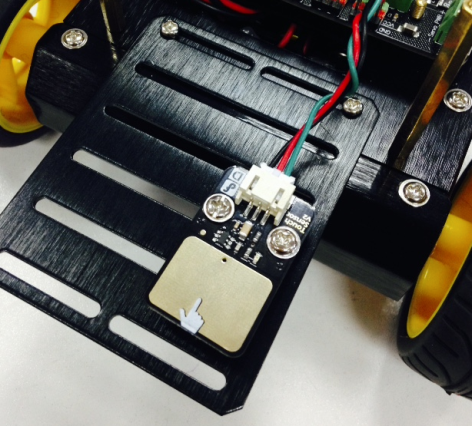
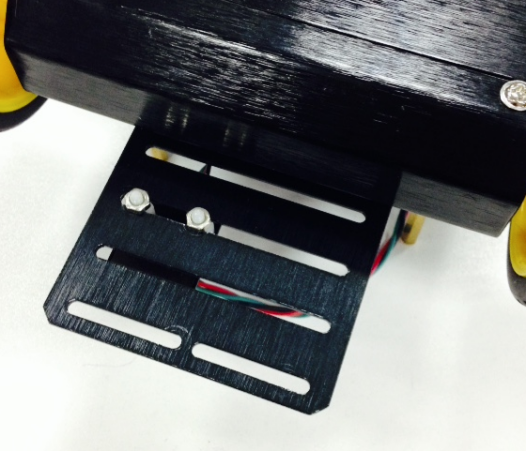
STEP2: Add the LCD Screen
Slide the shores into the four holes of the LCD screen and fix them. Cut the remaining part of the tie-wraps.
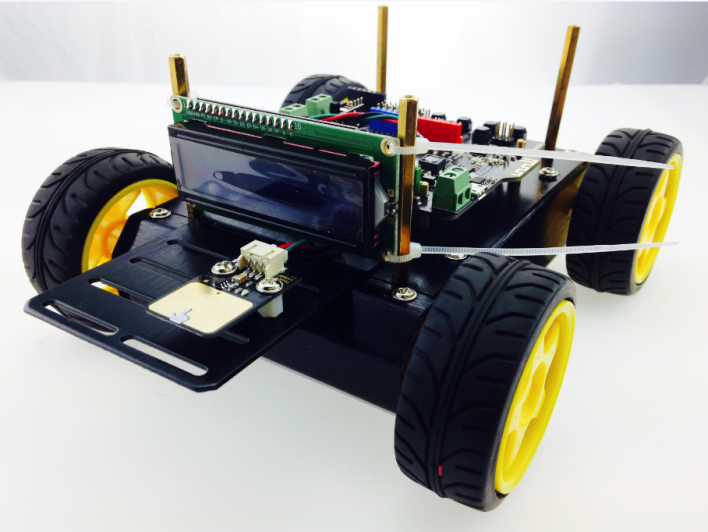
STEP3: Add the CO2 Sensor
Fix the Nylon columns on the CO2 Sensor. Attach the CO2 Sensor on the sensor plate.
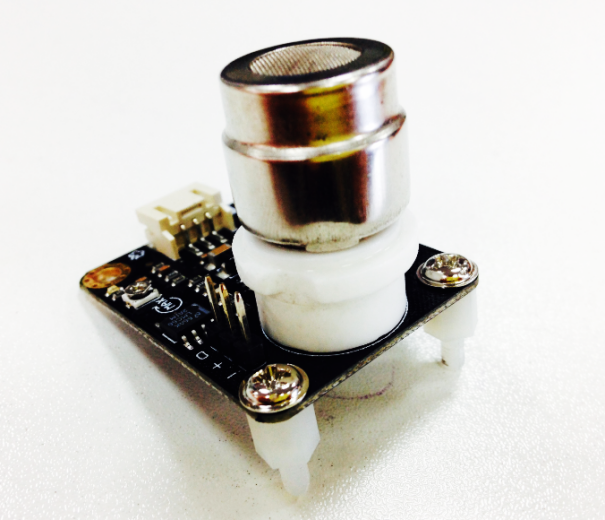
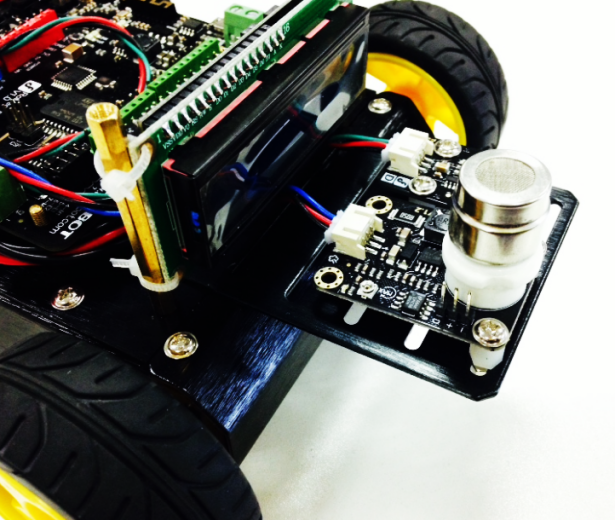
You almost complete the assembling. Please do not fix the upper plate onto the platform yet as we need to work on the circuit connection later.
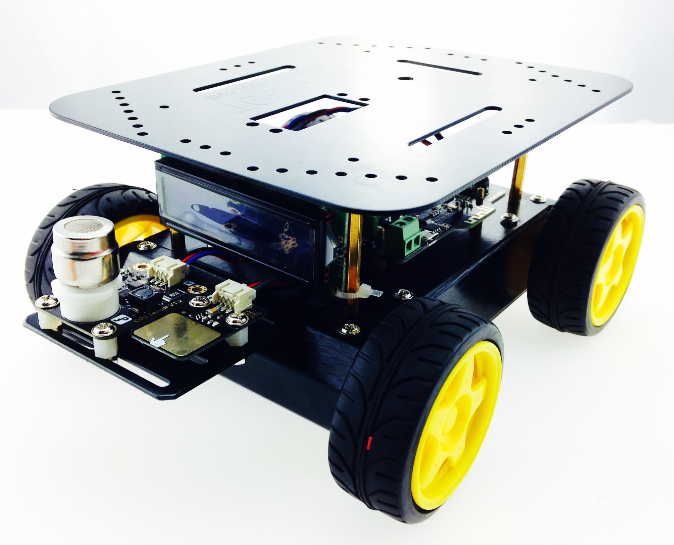
CONNECT THE HARDWARE:
Please keeping cables in order.
The interface is colored as follows:
Red indicates power
Black indicates ground
Blue indicates Analog Input Pin
Green indicates Digital I/O Pin
The LCD monitor should be connected to VCC, GND, SCL and SDA in that particular order.
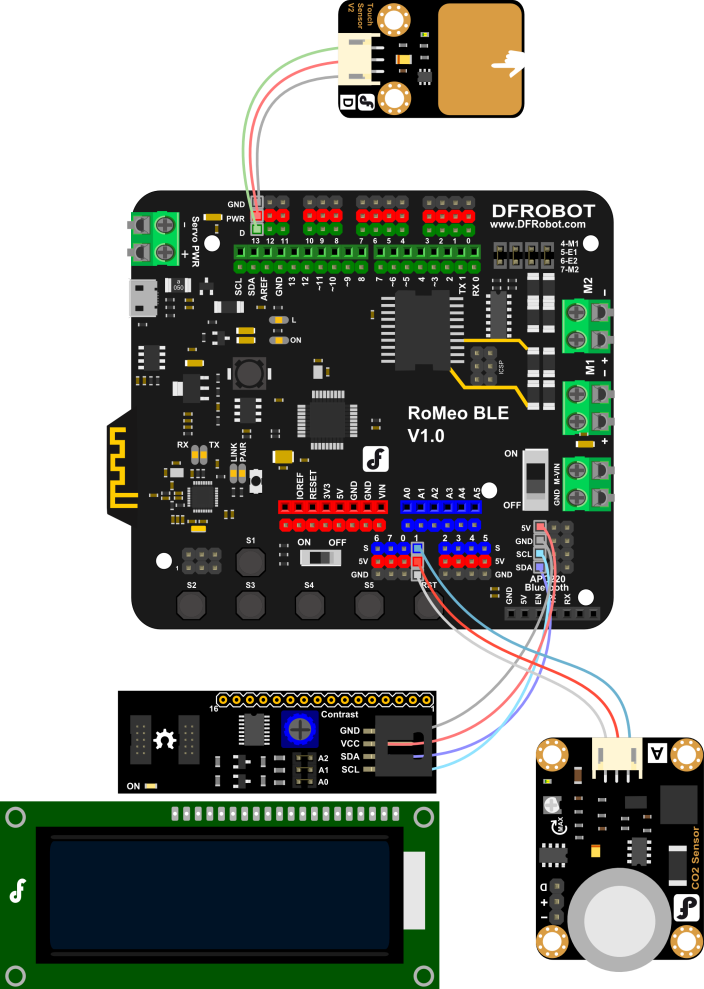
CODING
Find the code named DHT11_Display.ino and download it. Don’t forget the library for LiquidCrystal_I2C and CO2.
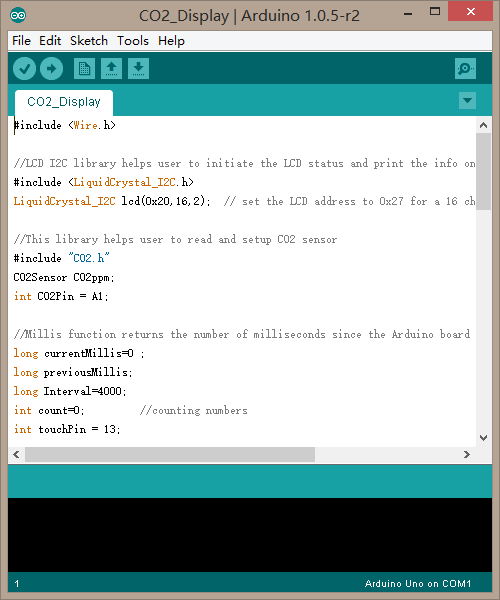
After successfully download the code, real-time CO2 density will be shown on the LCD screen. The touch sensor has two functions here:
1. If you don’t touch the sensor after some time, it will turn off automatically.
2. If you want to add more sensors to monitor other environmental data, the touch sensor can switch the monitoring data on the LCD.
CODE SYNOPSIS
Library is important. It is hard to understand the library without library.
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x20,16,2);
#include "CO2.h"
CO2Sensor CO2ppm;
Here you need to know about CO2Pin, a variable that is used to declare the sensor’s pins.
int CO2Pin = A1;
Namely, DHT11Pin represents Analog Pin1. That is to say, our CO2 sensor is connected to Analog Pin1.
The followings are some declarations for the time variables. TouchPin represents touch sensor while 13 stands for digital pin.
long currentMillis=0;
long previousMillis;
long Interval=4000;
int count=0; //counting numbers
int touchPin = 13;
Bring in the function of setup(), which is a setting-up for the initation.
pinMode(touchPin,INPUT);
Then keep the touch sensor with a type-in mode. For specific information, you may check the Arduino Reference in Arduino Website(www.arduino.cc), which has introductof the function of pinMode().
Next, you need to initialize the LCD screen and turn on the LCD light, which show that LCD screen is ready.
lcd.init();
lcd.backlight();
delay(100);
lcd.setBacklight(0);
Now it’s turn for the function of loop(). First we need to read the value from the touch sensor and then store those data with one variable touchState.
int touchState = digitalRead(touchPin);
Then check if the controller will receive a signal of HIGH once you touch the touch sensor with you fingers, 1 shall be added to the count.
if (touchState == HIGH){
count++;
previousMillis= millis();
}
Hereby count means how many times you have touched the screen. But if you only touch the sensor one time, then the amount of time for each touch will be included in the function of millis().
We change the length of touch time with a sub sentence initiating with if. Interval here means the period for touch we set up. Thus, we know what action shall be taken within four-second of touch and more than four seconds’ touch respectively.
if(currentMillis - previousMillis < Interval) {
//do something in 4 second
else{
//do something more than 4 seconds
}
lcd.setBacklight(0);
The function of setBacklight() is used to turn off the LCD backlight lamp.
What action shall be taken when we touch the sensor for more than four seconds
If we touch the sensor for more than four seconds, we know that the LCD backlight lamp can be turned off.
what action shall be taken within four-second of touch.
if (count==1){
// One touch, the LCD screen won’t show any difference
}
else if (count==2){
// Touch twice, value will be shown on the LCD screen
}
Press the touch sensor one more time within four seconds; the screen would still be off. Only if you touches it twice at the same time, will the LCD backlight be on and figures of CO2 density be shown.
Please remember to keep the count as zero after you touch the sensor for the last time.
count=0;
Thus the complete code shall be:
if (count==1){
lcd.setBacklight(0);
}
else if (count==2){
lcd.backlight();
DustShow();
count=0;
}
Then we need to keep a track of the current time as we can compare it with previousMillis. This point is very important.
currentMillis = millis();
The function of CO2ppm.Read() is used to read data. And the variable CO2Value will be used to store the data from the CO2 sensor.
int CO2Value=CO2ppm.Read(CO2Pin);
Here is how we would used the function related to LCD screen.
lcd.setCursor(0,0);
lcd.setCursor(0,1);
The function of setCursor(column,row) is used to demonstrate which column and row the cursor is shown, starting from zero within the brackets.
lcd.print(CO2Value);
print() means this figure can be shown on the screen directly.
lcd.print(" ");
lcd.print(" ") means blank space shown on the screen. It’s used to clear the screen.
A Combination of Multiple Sensors
How can you combine multiple environmental sensors once you have bought some kind of sensors?
Don’t worry. We will offer you guys a coding template for the testing of multiple sensors. You can make adjustments of the combination by referring to the mentioned template. In fact, the theory is the same as single sensor except that there are for steps for the changes of LCD screen.
The coding in red below needs to be modified. We mentioned before that count refers to how many times fingers touch the sensor. Thus, count=2 means that we have pressed twice and it shows the figures for the first sensor. Keep going! Please bear in your mind that you shall keep the count zero again.
Sample Code:
if(currentMillis - previousMillis < Interval) {
if (count==1){
lcd.setBacklight(0);
}
else if (count==2){ //No.1 Sensor
Sensor1Show();
lcd.backlight();
else if(count==3){ //No.2 Sensor
Sensor2Show();
lcd.backlight();
count = 0;
}
}
Of course, initiation set-up, declaration of variables at the beginning, for the sensor is important.
You can check the sample code named WeatherStation.ino for reference if you still have no idea how to modify your codes.