This project is made by LAGSILVA.
"Lottery Winner" for Arduino creates a sequence of non-repeating pseudo-random numbers for lottery or other applications.

Things used in this project
Hardware components
Software apps and online services
Arduino IDE
Story
1) Introduction
One of the first projects I have done by myself for Arduino was a random number generator.
That is good for beginners because the logic/code is very simple using basic Arduino functions.
But in this case, some numbers in a random sequence can be repeated and this works for a dice game, for example, but not for lotteries.
For this upgraded version I have introduced new features such as shuffling and sorting routines.
Now is possible to setup the range of numbers (from 1 to 99) and also the set of non-repeated draw numbers (from 1 to 10).
It is a complete (non repeating) pseudo-random number generator and very easy to assemble and to configure that can be used in other applications!
I hope you enjoy it and have fun!
Good luck in the lottery and don't forget to send me a donation if you win! ;-)
Cheers!
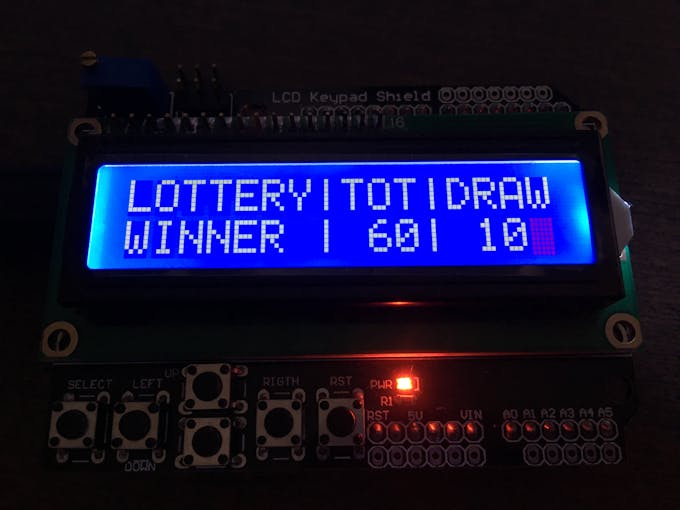
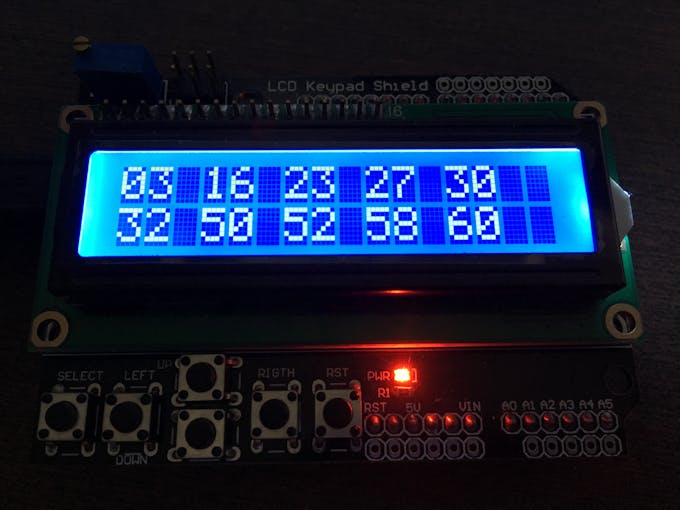
2) Shuffling
The main feature of this project is the shuffling routine that simulates the process of taking out the numbered balls from within a box.
A ball is randomly removed from the box and the process is repeated so many times as necessary to complete the sequence of draw numbers.
// Shuffling Routine
void shuffle() {
byte k, n;
String tempSeq = dezenas;
shuffleDezenas = "";
for (k = 0; k < maxSorteio; k++) {
n = random(tempSeq.length() / 2);
shuffleDezenas = shuffleDezenas + tempSeq.substring(n * 2 , n * 2 + 2);
tempSeq.remove(n * 2, 2);
}
}
I like working with 'strings' because there are some interesting functions available to manipulate them (take a look on String Object and String at Arduino's Reference page).
These fuctions simplify coding and speed up the development process.
The most important function for this project is the string.remove, because it simulates the action of removing the ball inside a box.:
string.remove(index, count)
Parameters
index: a variable of type unsigned int
count: a variable of type unsigned int
3) Sorting
The sorting routine is important to organize the set of draw numbers in crescent order.
This routine scans all numbers and selects the minor of them. This number is removed and placed in another list. The scanning process is repeated until there are no more numbers remaining in original list.
The process is similar to shuffling, but this time the number removed is not randomly selected.
// Sorting routine
void sort() {
String tempSeq, c;
byte i, k, n, menorDezena, menorPos;
sortDezenas = "";
tempSeq = shuffleDezenas;
menorDezena = maxDezenas;
do {
for (k = 0; k < tempSeq.length() / 2; k++) {
n = (tempSeq.substring(k * 2 , k * 2 + 2)).toInt();
if (n <= menorDezena) {
menorDezena = n;
menorPos = k;
c = tempSeq.substring(k * 2 , k * 2 + 2);
}
}
tempSeq.remove(menorPos * 2, 2);
sortDezenas = sortDezenas + c;
menorDezena = maxDezenas;
} while (tempSeq.length() > 0);
}
4) Operation
The operation is very simple using the keypad of the shield.
UP button: Increases by 1 the range of draw numbers (Max. is 99).
DOWN button: Decreases by 1 the range of draw numbers (Min. is 1).
RIGHT button: Increases by 1 the draw numbers (Max. is 10).
LEFT button: Decreases by 1 the draw numbers (Min. is 1).
SELECTbutton: Generates the draw numbers.
During the setup the LCD shows the information about the total (TOT) range and the set of draw numbers (DRAW).
After the SELECT button is pressed, the numbers of the draw will appear sorted on the LCD after a series of repetitions, causing a certain suspense before the result.
/* Project: LOTTERY WINNER
Hardware: Arduino UNO-R3
Author: LAGSILVA
Date: 21.Sep.2018
Rev: V1.0
Revision Notes:
1) Shuffling & Sorting routines
2) Setup of total numbers (10 to 99) and total drawn (1 to 10)
*/
#include <LiquidCrystal.h>
LiquidCrystal lcd(8, 9, 4, 5, 6, 7);
String shuffleDezenas, sortDezenas, dezenas;
byte maxSorteio = 6, maxDezenas = 60;
byte botoes = 5;
int estadoBotoes = 0;
#define btnRight 1
#define btnLeft 2
#define btnUp 3
#define btnDown 4
#define btnSelect 5
#define btnNone 6
void setup() {
byte k;
randomSeed(analogRead(1));
lcd.begin(16, 2);
lcd.clear();
tela();
criarDezenas();
}
void loop() {
byte k;
botoes = ler_botoes();
if (botoes == btnRight) {
maxSorteio = constrain(maxSorteio + 1, 1, 10);
tela();
}
if (botoes == btnLeft) {
maxSorteio = constrain(maxSorteio - 1, 1, 10);
tela();
}
if (botoes == btnUp) {
maxDezenas = constrain(maxDezenas + 1, 10, 99);
criarDezenas();
tela();
}
if (botoes == btnDown) {
maxDezenas = constrain(maxDezenas - 1, 10, 99);
criarDezenas();
tela();
}
if (botoes == btnSelect) {
lcd.clear();
for (byte rep = 1; rep <= 9; rep++) {
shuffle();
delay(100);
lcd.setCursor(0, 0);
for (k = 1; k <= maxSorteio / 2; k++) {
lcd.print(shuffleDezenas.substring((k - 1) * 2, (k - 1) * 2 + 2));
lcd.print(" ");
}
lcd.setCursor(0, 1);
for (k = maxSorteio / 2 + 1; k <= maxSorteio; k++) {
lcd.print(shuffleDezenas.substring((k - 1) * 2, (k - 1) * 2 + 2));
lcd.print(" ");
}
}
sort();
lcd.setCursor(0, 0);
for (k = 1; k <= maxSorteio / 2; k++) {
lcd.print(sortDezenas.substring((k - 1) * 2, (k - 1) * 2 + 2));
lcd.print(" ");
}
lcd.setCursor(0, 1);
for (k = maxSorteio / 2 + 1; k <= maxSorteio; k++) {
lcd.print(sortDezenas.substring((k - 1) * 2, (k - 1) * 2 + 2));
lcd.print(" ");
}
}
}
int ler_botoes() { // Buttons reading
delay(120);
estadoBotoes = analogRead(0);
if (estadoBotoes > 1000) return btnNone;
if (estadoBotoes < 50) return btnRight;
if (estadoBotoes < 250) return btnUp;
if (estadoBotoes < 450) return btnDown;
if (estadoBotoes < 650) return btnLeft;
if (estadoBotoes < 850) return btnSelect;
return btnNone; // Return NONE when all options fails
}
void shuffle() { // Shuffling routine
byte k, n;
String tempSeq = dezenas;
shuffleDezenas = "";
for (k = 0; k < maxSorteio; k++) {
n = random(tempSeq.length() / 2);
shuffleDezenas = shuffleDezenas + tempSeq.substring(n * 2 , n * 2 + 2);
tempSeq.remove(n * 2, 2);
}
}
void sort() { // Sorting routine
String tempSeq, c;
byte i, k, n, menorDezena, menorPos;
sortDezenas = "";
tempSeq = shuffleDezenas;
menorDezena = maxDezenas;
do {
for (k = 0; k < tempSeq.length() / 2; k++) {
n = (tempSeq.substring(k * 2 , k * 2 + 2)).toInt();
if (n <= menorDezena) {
menorDezena = n;
menorPos = k;
c = tempSeq.substring(k * 2 , k * 2 + 2);
}
}
tempSeq.remove(menorPos * 2, 2);
sortDezenas = sortDezenas + c;
menorDezena = maxDezenas;
} while (tempSeq.length() > 0);
}
void criarDezenas() { // Numbers list creation routine
byte k;
dezenas = "";
for (k = 1; k <= maxDezenas; k++) {
if (k < 10) {
dezenas = dezenas + "0" + k;
}
else {
dezenas = dezenas + k;
}
}
}
void tela() { // LCD print routine
lcd.setCursor(0, 0);
lcd.print("LOTTERY|");
lcd.setCursor(0, 1);
lcd.print("WINNER |");
lcd.setCursor(8, 0);
lcd.print("TOT|DRAW");
lcd.setCursor(9, 1);
lcd.print(maxDezenas);
lcd.print("| ");
if (maxSorteio < 10) {
lcd.print("0");
lcd.print(maxSorteio);
}
else {
lcd.print(maxSorteio);
}
}