The objective of this ESP32 / ESP8266 MicroPython Tutorial is to explain how to read a file from MicroPython’s file system. This tutorial was tested on both the ESP32 and the ESP8266.
Introduction
The objective of this ESP32 / ESP8266 MicroPython Tutorial is to explain how to read a file from MicroPython’s file system. This tutorial was tested on both the ESP32 and the ESP8266. The prints shown were taken from the tests on the ESP8266.
Usually, I use Putty to interact with the MicroPython prompt. Nevertheless, just for demonstration purposes, I’m going to use the Arduino serial monitor to interact with the devices in this post. Note that since it can establish a serial connection with a device, it works the same way it would on Putty.
To use it, we just need to select the correct COM port and, when the serial monitor opens, the correct baud rate, which is 115200. Note that we also need to choose Carriage return or Both NL & CR on the dropdown on the left of the baud rate, so the prompt knows that a command as ended.
Nevertheless, you can keep using Putty or other software of your choice to interact with the devices if you prefer.
The code
First os all, we will create a new file in MicroPython’s file system. If you need a detailed tutorial on how to do it, please check this previous post. We will create a file named initialFile.txt and write two lines of text on it. Then we will close the file and confirm that it was created by listing the files on the current directory.
file = open ("initialFile.txt", "w")
file.write("First Line \n")
file.write("Second Line")
file.close()
import os
os.listdir()
You should get an output similar to figure 1 after running the code.

Figure 1 – Writing the initial file to the file system.
Now, to open the file, we will use the same open function, passing as first argument the name of the file, but now we pass as second the string “r“, indicating that we want to read its content.Then, to read the whole file content, we just call the read method on the file object. As input, this method can receive a number of bytes to be read from the file. If no argument is given, it will read the whole content until the end of the file.
We are going to perform our test by calling the method without any arguments, so the whole file content is returned. After the call, you should get the content printed. Note that the newline (“\n”) character it will get explicitly printed.
Finally, close the file with a call to the close method.
file = open("initialFile.txt", "r")
file.read()
file.close()
We can repeat the previous test but instead of calling the read method, we will call the readline method, which returns a line of the file. Note that this method can receive a maximum number of characters to read from the line, and it stops after that number of characters is received or the end of the line is reached. If we don’t specify any argument, it will read an entire line. Since we have two lines, we will call it twice, with no arguments.
Note that this call will return an empty string if we already reached the end of the file. So, if we didn’t now the number of lines beforehand, this could be used as a stopping condition.
As we did before, in the end we must close the file with the call to the close method.
file = open("initialFile.txt", "r")
file.readline()
file.readline()
file.close()
The expected result for running both groups of commands is shown bellow.

Figure 2 – Result of reading the file with two different approaches.
Note from figure 2 that the readline method also prints the “\n” character. If we want to get rid of it, we can call the rstrip method on the returned string, which receives as input a string with a set of characters to be removed from the right. If they are not found, nothing happens. So, we can call this method passing as argument the “\n” char.
file = open("initialFile.txt", "r")
file.readline().rstrip("\n")
file.readline().rstrip("\n")
file.close()
Check bellow at figure 3 the result. Note that the “\n“ character no longer appears. The space at the end of the first line is correct since in the original string I’ve added an empty space before the “\n“.

Figure 3 – Removing the “\n” character at the end of the string.
To avoid leaving garbage in our file system, we will remove the file by calling the remove function of the os module, passing as input the name of the file. If we then call the listdir function, the file should no longer be listed.os.remove("initialFile.txt")
os.listdir()
At figure 4 there’s the output of this command. As can be seen, only the boot.py remains. Don’t remove that file.
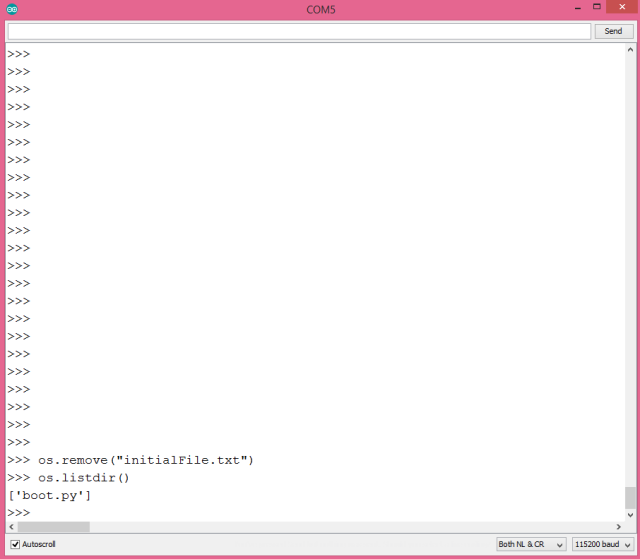
Figure 4 – Removing a file from MicroPython’s file system.
Final note
As a final note, I will leave a useful way of checking the methods and functions available from objects. To do so, we just need to call the dir function and pass as input the object from which we want to get the list.
dir(os)
dir(file)
dir(str)
We should get a list as shown in figure 5, which contains the methods available for some objects. This is very useful when we don’t find documentation.
Figure 5 – Listing the methods from the objects.