Declaring variables is a useful way of naming and storing values for later use by the program. Integers (int) represent numbers ranging from -32768 to 32767.
In the above example, we have input an integer: 10. “LedPin” is the variable name we have chosen. Of course, you may it anything you like instead of ledPin, but it's better to name the variable according to its function. Here the variable ledPin indicates that the LED is connected to Pin-out 10 of Arduino.
Use a semicolon (;) to conclude the declaration. If you don’t use the semicolon, the program will not recognise the declaration, so this is important!
What is a variable?
A variable is a place to store a piece of data. It has a name, a value, and a type. In the above example, “int” (integer) is the type, “ledPin” is the name and “10” is the value. In this example we’re declaring a variable named ledPin of type “int” (integer), meaning the LED is connected to digital pin 10. Variables display as orange text in the sketch.
Integers can store numbers from -32768 to 32767. You must introduce, or declare variables before you use them. Later on in the program, you can simply type the variable name rather than typing out the data over and over again in each line of code, at which point its value will be looked up and used by the IDE.
When you try to name a variable, start it with a letter followed with letter, number or underscore. The language we are using (C) is case sensitive. There are certain names that you cannot use such as “main”, “if”, “while” as these have their own pre-assigned function in the IDE.
Don’t forget the variable names are case-sensitive!
The setup() function
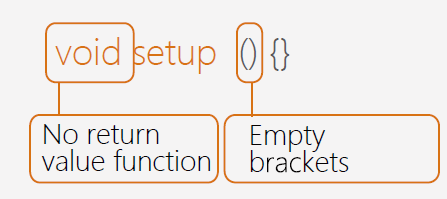
The setup() function is read by the Arduino when a sketch starts. It is used it to initialize variables, pin modes, initialize libraries, etc. The setup function will only run once after each power-up or reset of the Arduino board.
In this example there is only one line in the setup() function:
pinMode
pinMode(ledPin, OUTPUT);
This function is used to define digital pin working behavior. Digital pins are defined as an input (INPUT) or an output (OUTPUT). In the example above you can see brackets containing two parameters: the variable (ledPin) and its behaviour (OUTPUT).
“pinMode” configures the specified digital pin to behave either as an input or an output. It has two parameters:
“pin”: the number of the pin whose mode you wish to set
“mode”: INPUT, OUTPUT, or INPUT_PULLUP.
If you want to set the digital pin 2 to input mode, what code would you type?
Function
Segmenting code into functions allows a programmer to create modular pieces of code that perform a defined task and then return to the area of code from which the function was "called". The typical case for creating a function is when one needs to perform the same action multiple times in a program.
There are two required functions in an Arduino sketch, setup() and loop(). Other functions must be created outside the brackets of those two functions.
Difference of INPUT and OUTPUT
INPUT is signal that sent from outside events to Arduino such as button. OUTPUT is signal that sent from Arduino to the environment such as LED and buzzer.
If we scroll further down, we can see the main part of the code. This is the loop.
void loop() {
digitalWrite(ledPin,HIGH);
delay(1000);
digitalWrite(ledPin,LOW);
delay(1000);
}
The Arduino program must include the setup () and loop () function, otherwise it won t work.
After creating a setup() function, which initializes and sets the initial values, the loop() function does precisely what its name suggests, and loops consecutively, allowing your program to change and respond. Use it to actively control the Arduino board. Here we want to control the LED constantly on and off every other second. How can we make that happen?
In this project we want the LED to turn on for 1 second and then turn off for 1 second, and repeat this action over and over. How can we express this in code?
Look at the loop () function within the first statement. This involves another function: digitalWrite ().
digitalWrite(ledPin,HIGH);
The function format is as follows:
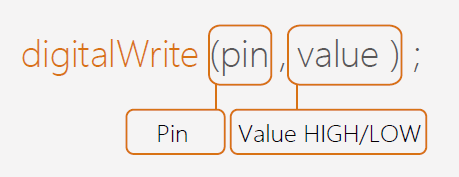
digitalWrite writes a HIGH (on) or a LOW (off) value to a digital pin. If the pin has been configured as an OUTPUT with pinMode(), its voltage will be set to the corresponding value: 5V (or 3.3V on 3.3V boards) for HIGH and 0V (ground) for LOW. Please note that digitalWrite() is applied only under the condition that pinMode() is set as OUTPUT. Why? Read on!
The Relation of pinMode(), digitalWrite() and digitalRead()
If pinMode configures a digital pin to behave as an input, you should use the digitalRead() function. If the pin is configured as an output, then you should use digitalWrite().
NOTE: If you do not set the pinMode() to OUTPUT, and connect an LED to a pin, when calling digitalWrite(HIGH), the LED may appear dim. This is because without explicitly setting a pin-Mode(), digitalWrite() will enable the internal pull-up resistor, which acts like a large current-limiting resistor.
Next:
delay(1000);
delay() pauses the program for the amount of time specified (in miliseconds). (There are 1000 milliseconds in 1 second.)
Hardware
Breadboard
Breadboard is a way of constructing and testing circuits without having to permanently solder them in place. Components are pushed into the sockets on the breadboard and then extra "jumper" wires are used to make connections.
The breadboard has two columns, each with 17 strips of connections. In this diagram the black lines show how the top row is connected. This is the same for every other row. The two columns are isolated from one-another.
Resistors
As the name suggests, resistors resist the flow of electricity. The higher the value of the resistor, the more resistance it has and the less electrical current will flow through it. The unit of resistance is called the Ohm, which is usually shortened to Ω( The geek letter Omega).
Unlike LEDs, resistors are not polarized (do not have a positive and negative lead) - they can be connected either way around. Normally a LED needs 2V of voltage and 35 mA current to be lit, so with a resistor of would be able to control the flow you might risk burning it out. Be careful of this because resistors can get hot!
If you want to read the resistance value from the resistor color code, visit this website for calculation tables: http://www.21ic.com/tools/compo nent/201003/54192.htm
Read Resistor Color Rings
The resistor value will be marked on the on the outer package of your resistors, but what should we do in case the label gets lost and there are no measuring tools at hand? The answer is to read the resistor value. This is a group of colored rings around the resistor. Details are available online for those who are interested in having a try.
Here is an online calculator for five-color-ring resistor value calculating: http://www.21ic.com/tools/compo nent/201003/54192.htm
LEDs
A light-emitting diode (LED) is a two-lead semiconductor light source. It is basic pnjunction diode, which emits light when activated.
Typically, LEDs have two leads, one positive and one negative. There are two ways to tell which is the positive lead of the LED and which the negative: Firstly, the positive lead is longer. Secondly, where the negative lead enters the body of the LED, theremis a flat edge to the case of the LED.
LEDs are polarized, so they have to be connected the right way around. If you put the negative lead of an LED in to the power supply and the positive lead to ground, the component will not wor , as can be seen in the diagram to the right.
In your kit, you can also find LEDs with 4 leads. This is an RGB LED with 3 primary color LEDs embedded in to it. This will be explored later.